Types of data in JavaScript
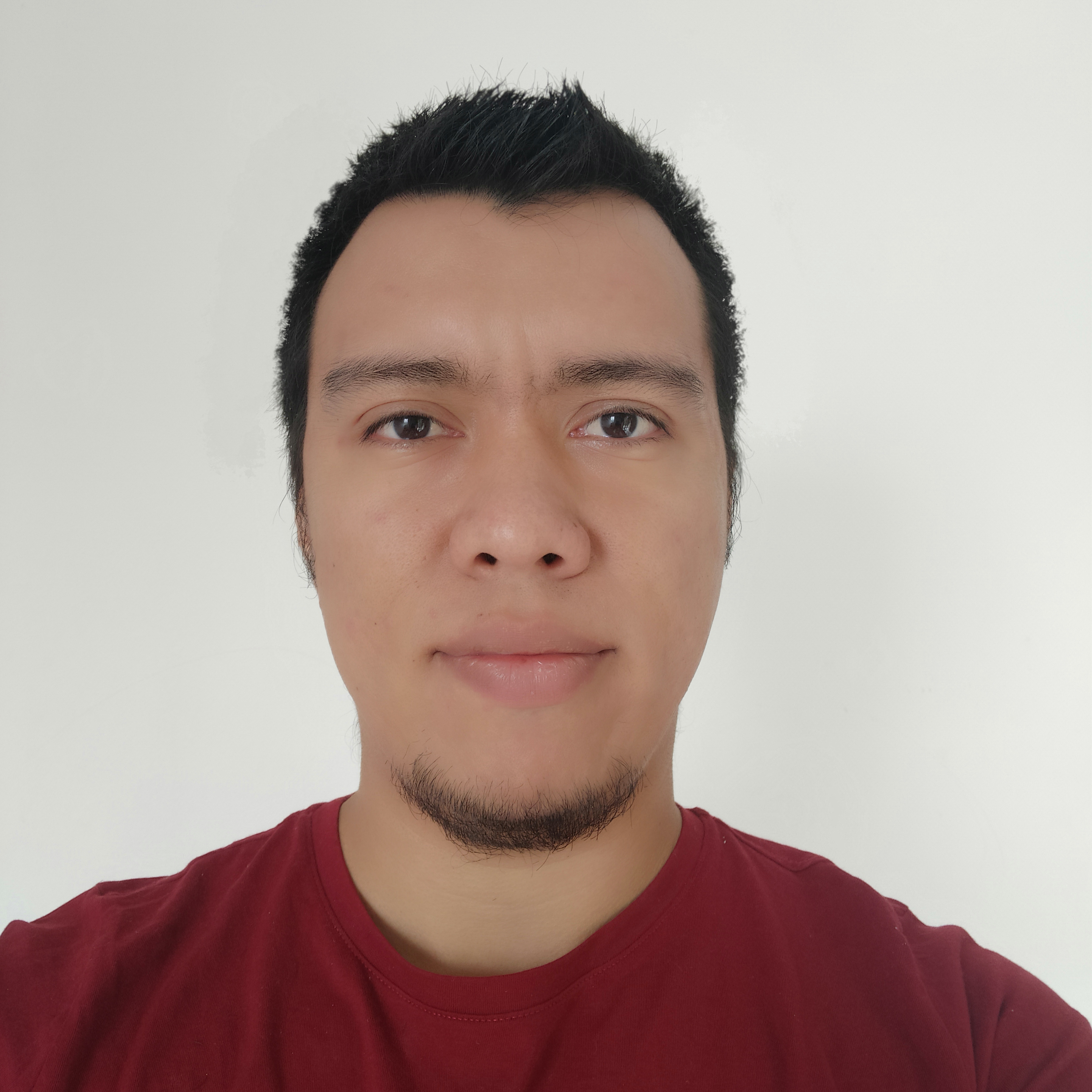
In JavaScript there are basically six primitive data types, objects and functions. In addition to this, there is a special type of primitive data called null and a type added in ECMA 2015 called Symbol. Next, we will see how to identify each of these data types and some very simple examples.
Primitive types
Primitive types are a data types that store a single value. Among these data types we have: undefined, Boolean, Number, String, BigInt, Symbol and even null which is a special type.
Type | Description | Value |
---|---|---|
Undefined | Undefined Variables | undefined |
Boolean | Logical entity of two values | false true |
Null | Absence of an object value | null |
Number | Integers | a number |
BigInt | Integers (large range) | a large number |
String | Character strings | a string |
In the following examples we use typeof to see the specific data type in Javascript.
typeof undefined // undefined
typeof true // "boolean"
typeof null // "object"
typeof 40 // "number"
typeof 12n // "bigInt"
typeof "hello world" // "string"
Note that the value null is actually an object.
Objects
An object represents structured data and instructions for working with that data. It is a collection of key-value pairs where the values can be other data types. Let's see the following example.
{
model: 'Mazda',
year: 2020
}
However, arrays are also objects.
typeof [1,2,3] // "object"
Functions
Functions are pieces of code that can be called from other parts of the code.
function foo() {
return 'Hello';
}
foo() // 'Hello'
typeof foo // "function"
Like the other types, it is possible to obtain the specific type with the typeof operator.
typeof foo // "function"
Symbols
Symbols are sets of non-string values. They are usually used to create unique keys in objects, among other uses.
let id = Symbol("id");
let user = {
name: "John",
[id]: 123
}
let id2 = Symbol("id");
user[id2] = 100;
user[id]; // 123
user[id2]; // 100
To obtain the data type, we can also use the typeof operator.
typeof id // "symbol"