What is Eloquent in Laravel?
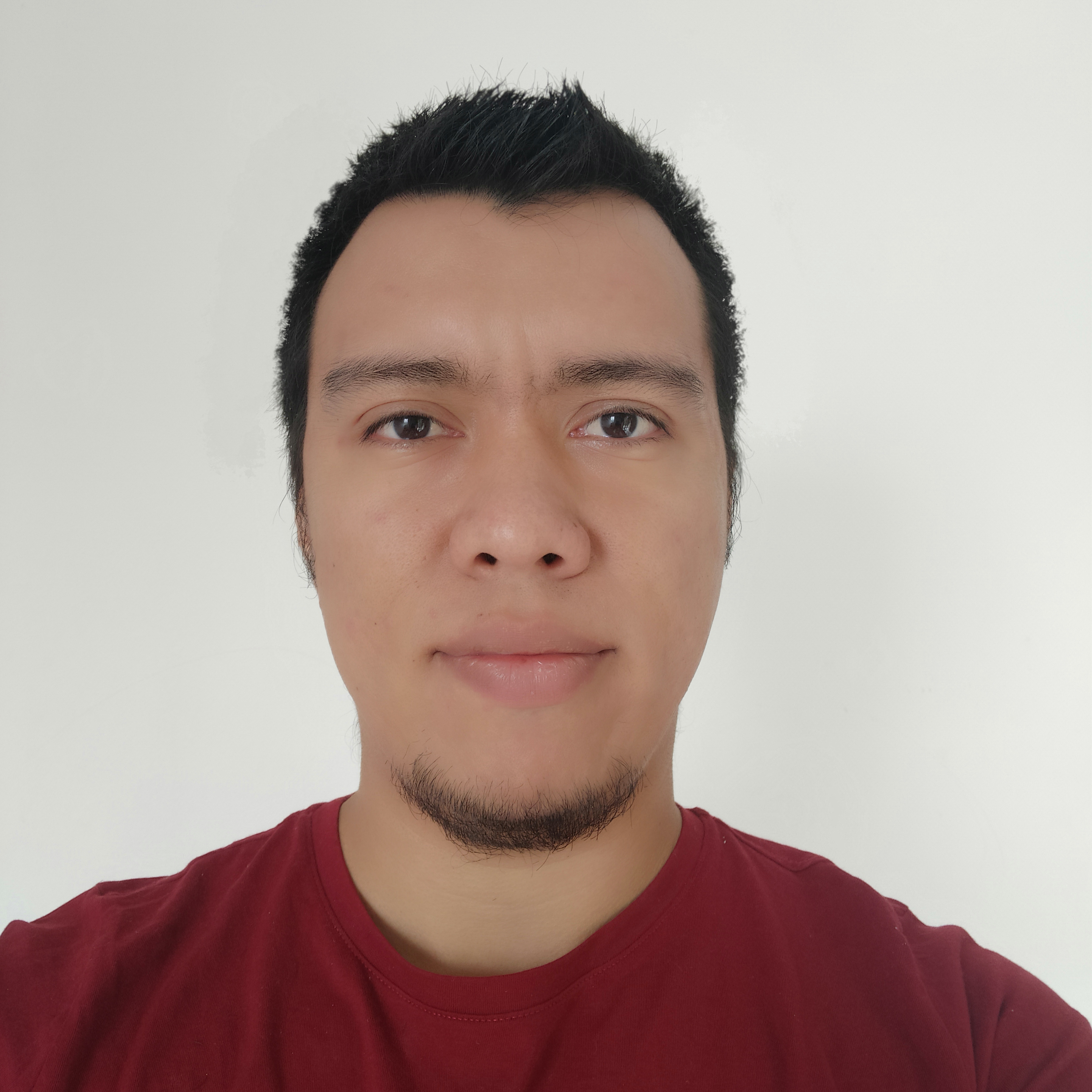
Eloquent is the laravel ORM that implements the Active Record architecture pattern. That means that each table in the database corresponds to a PHP class (model) that interacts with it. Each instance of such class corresponds to a record in the table and generates persistence in the database, which means that an update of the class attributes can alter the corresponding record in the database.
Active Record
To make the Active Record implementation a bit clearer, let's create the following table by means of a migration.
php artisan make:migration create_employees_table
If you don't know what a migration is, we recommend you to read our post Database migrations in Laravel. Let's define the structure of the employees table in the CreateEmployeesTable
model.
use Illuminate\Support\Facades\Schema;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Database\Migrations\Migration;
class CreateEmployeesTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('employees', function (Blueprint $table) {
$table->bigIncrements('id');
$table->string('dni');
$table->string('name');
$table->string('surname');
$table->integer('age');
$table->decimal('salary', 8, 2);
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('employees');
}
}
Let's execute the migration, create the model and enter laravel's tinker. Tinker is an interactive shell that comes as a dependency in the Laravel installation, where we can execute PHP commands and interact very easily with the classes, models and other elements of our application.
php artisan migrate
php artisan make:model Employee
php artisan tinker
Let's use the new model we just generated and create a new record in the database. Notice that for each command executed in the console, tinker returns the expression's value.
Psy Shell v0.9.9 (PHP 7.2.5 — cli) by Justin Hileman
>>> $employee = new \App\Employee;
=> App\Employee {#2960}
>>> $employee->id = 1;
=> 1
>>> $employee->dni = '1110355987';
=> "1110355987"
>>> $employee->name = 'John';
=> "John"
>>> $employee->surname = 'Doe';
=> "Doe"
>>> $employee->age = 25;
=> 25
>>> $employee->salary = 2300;
=> 2300
>>> $employee->save();
=> 2300
The last used method (save
) performs the persistence in the database by saving the record. To verify that it has indeed been saved, we can use eloquent's find
method as follows:
>>> (new \App\Employee)->find(1)
=> App\Employee {#2965
id: 1,
dni: "1110355987",
name: "John",
surname: "Doe",
age: 25,
salary: "2300.00",
created_at: "2019-07-13 05:00:57",
updated_at: "2019-07-13 05:00:57",
}
This method performs the search for the record directly in the database by its primary key. If you're as curious as I am and have wondered how laravel has managed to perform a bind of our model and the table in the database, it is because laravel generally uses singular names for models and assumes plural names for tables in the database. That is, our Employee
model is related to the Employees table. In the previous example we created the model separately, but there's a command in laravel that creates a migration and its associated model all in one step. This way we save ourselves the trouble of using the make:migration command and have the following equivalent command instead.
php artisan make:model Employee --migration
Or,
php artisan make:model Employee -m
If you had executed this command, you would have seen that it creates the model with its singular name and the migration with its plural name. Like this, there are many other conventions used by Eloquent to make things a little easier for us. You can see Eloquent's conventions in the following post.
Eloquent's conventions in Laravel
Now that you know what Eloquent in Laravel is and have seen how it works in a nutshell, you're ready to perform CRUD operations on the database. In our next post we'll see how to interact with the database to perform basic operations on it. See you next time.