Controllers in Laravel
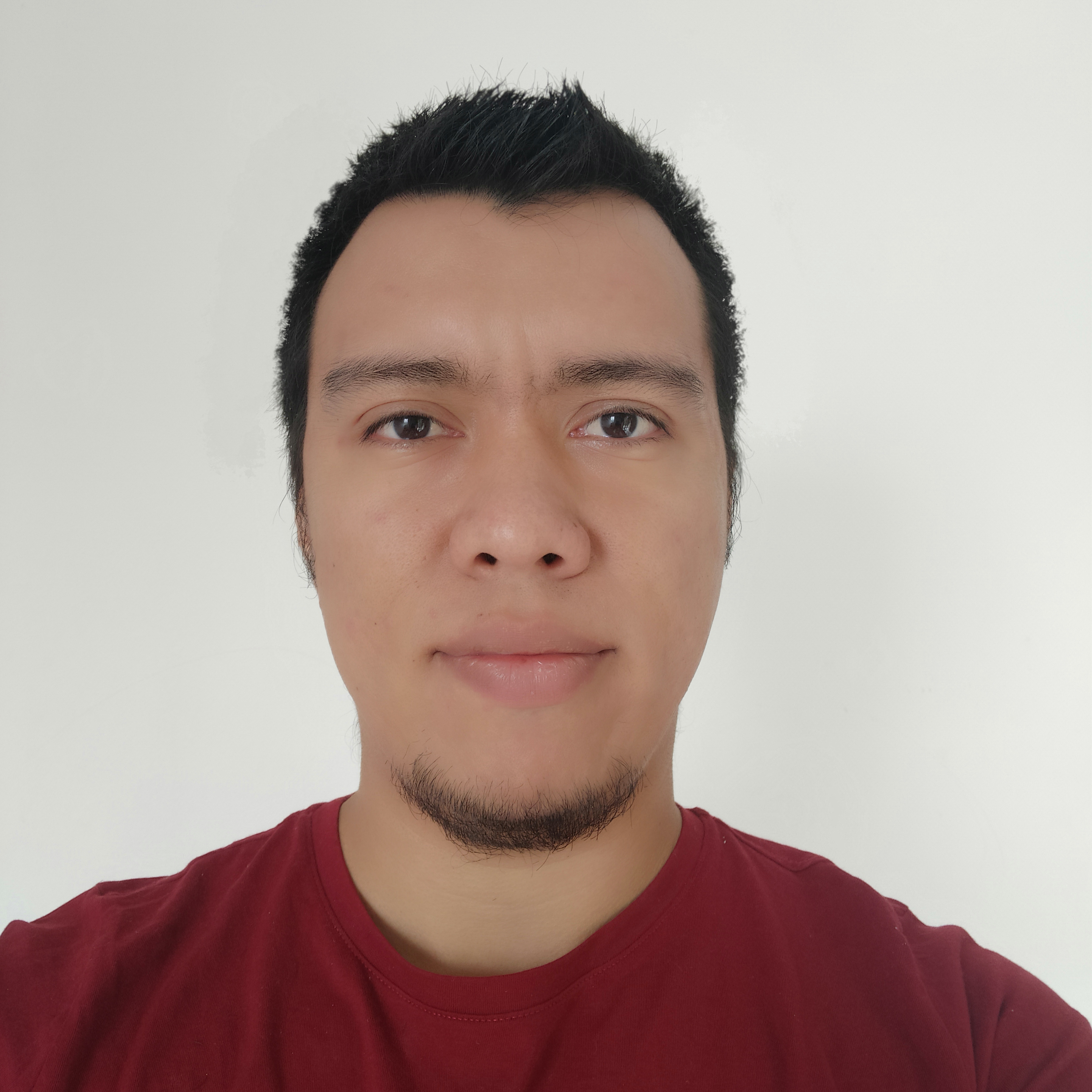
In one of our first posts we explained how the MVC pattern is implemented in laravel, today we will delve a little deeper into the component of the controllers. I highly recommend you have a clean laravel project so you can try the concepts learned here. Remember that the human brain learns 80% by doing and only 20% by reading (according to research). If you have already read our Laravel Installation post, you can start a clean project with the following command:
laravel new myProject
Basic Controllers
To create a controller, you must execute the following command with artisan.
php artisan make:controller BasicController
This command will create a controller called BasicController in the app/Http/Controllers folder with the following content:
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class BasicController extends Controller
{
//
}
Now, let's create the following method that will display a homepage or start page on our application.
public function index()
{
return view('home');
}
Finally, we will add the following line to our routes file in routes/web.php.
Route::get('/inicio', 'BasicController@index');
With this, we have defined the /inicio route in our application, which when accessed from the browser will execute the index
method of the BasicController controller. As you can see, it is enough to pass the name of the controller as the second parameter of the get
method or any other HTTP verbs, followed by the "@" character and the name of the method to be executed. Of course, if you return a view from said method you will need to create the view file. In our case, the file to create would be home.blade.php in the resources/views folder.
Namespaced Controllers
To create a controller inside a namespace whether it exists or not, you must run the following command with artisan:
php artisan make:controller User/ProfileController
For this particular case, the ProfileController.php file will be created inside app/Http/Controllers/User. If the User folder does not exist, it will be created automatically. Let's observe the code of the controller created:
namespace App\Http\Controllers\User;
use Illuminate\Http\Request;
use App\Http\Controllers\Controller;
class ProfileController extends Controller
{
//
}
As you can see, our controller has been placed in the expected namespace. To define it in a route, just change the slash to backslash as shown below.
Route::get('/myroute', 'User\ProfileController@index')
Single Method Controllers
On some occasions, it is necessary to create controllers that have only one method. It is then much more practical to use the __invoke
method which is executed when the controller is invoked. To create a controller with these characteristics, you must run the following command:
php artisan make:controller FrontController --invokable
As you can see, only the --invokable parameter has been added, which will create a controller similar to the following:
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class FrontController extends Controller
{
/**
* Handle the incoming request.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function __invoke(Request $request)
{
//
}
}
Although the scaffolding of this controller is designed to handle a request, note that any controller could have this same behavior. To simplify the example a little more, let's assume that this controller only handles a homepage or start page. We will then modify our controller as follows:
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class FrontController extends Controller
{
public function __invoke()
{
return view('home');
}
}
Finally, the route definition for this controller would look like this:
Route::get('/inicio', 'FrontController');
As you can see, it is not necessary to define any method since our controller uses the __invoke
method. See you soon!