Conventions of Eloquent in Laravel
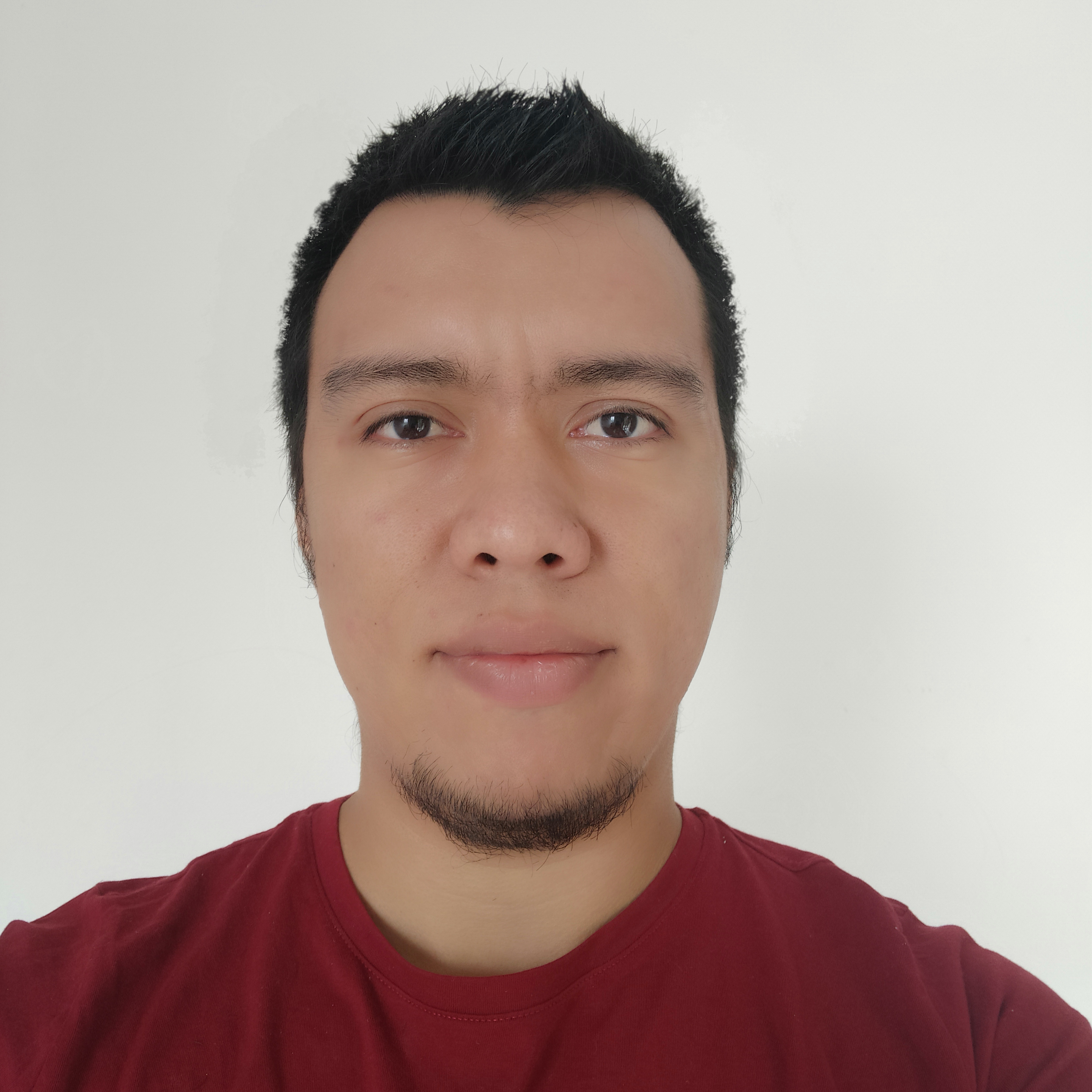
When starting with Laravel it is very easy to forget the default behavior regarding Eloquent. In this post we will enumerate some rules that Laravel assumes will facilitate your life. If you are here and you do not know what Eloquent is, we recommend you to read our post What is Eloquent in Laravel.
Model Conventions
( 1 ) The make:model command creates a file with the model name in the app folder. All models extend the Illuminate\Database\Eloquent\Model
class. Observe the file App/Empleado.php created with Artisan.
php artisan make:model Empleado
namespace App;
use Illuminate\Database\Eloquent\Model;
class Empleado extends Model
{
//
}
( 2 ) If you create a model with a singular name, Eloquent will assume by default that it is related to a table with the same name in plural. To change this behavior, simply specify the table name directly in the model class.
namespace App;
use Illuminate\Database\Eloquent\Model;
class Empleado extends Model
{
/**
* The table associated with the model.
*
* @var string
*/
protected $table = 'erp_empleados';
}
( 3 ) Eloquent assumes that the primary key name of a table is always id and that it is an incremental integer. Once again, to change this behavior, simply enter the model code and make the respective changes.
namespace App;
use Illuminate\Database\Eloquent\Model;
class Empleado extends Model
{
/**
* The primary key associated with the table.
*
* @var string
*/
protected $primaryKey = 'empleado_id';
/**
* Indicates if the IDs are auto-incrementing.
*
* @var bool
*/
public $incrementing = false;
/**
* The "type" of the auto-incrementing ID.
*
* @var string
*/
protected $keyType = 'string';
}
Use $keyType
only if the data type of the primary key column is not an integer.
( 4 ) Eloquent uses snake case by default for table names. Which means that compound names will be separated by the character _
(underscore).
Relationships
Relationships are defined with hasOne
or hasMany
.
return $this->hasOne('class', 'foreign_key', 'local_key');
( 5 ) Eloquent determines the foreign key of a relationship based on the model name followed by the name of the first key of the class where the relationship is defined.
namespace App;
use Illuminate\Database\Eloquent\Model;
class File extends Model
{
protected $primaryKey = 'idx';
public function fileDownloads()
{
return $this->hasOne(FileDownload::class);
}
}
This relationship will search for the file_idx column in the FileDownload
model's table.
( 6 ) Eloquent determines the local key of a relationship based on the model's primary key.
Following the previous example, the relationship will search for the local key idx in the File
model's table.
Inverse Relationships
Inverse relationships are defined with belongsTo
.
$this->belongsTo('class', 'foreign_key', 'local_key');
( 7 ) Eloquent determines the foreign key of an inverse relationship based on the method name and the primary key of the related table.
namespace App;
use Illuminate\Database\Eloquent\Model;
class File extends Model
{
protected $primaryKey = 'fileid';
}
namespace App;
use Illuminate\Database\Eloquent\Model;
class FileDownload extends Model
{
public function filex()
{
return $this->belongsTo(File::class);
}
}
This relationship will search for the filex_fileid column in the FileDownload
model's table.
( 8 ) Eloquent determines the local key of an inverse relationship based on the primary key of the related model.
Following the previous example, the relationship will search for the local key fileid in the File
model's table.