Functions in TypeScript
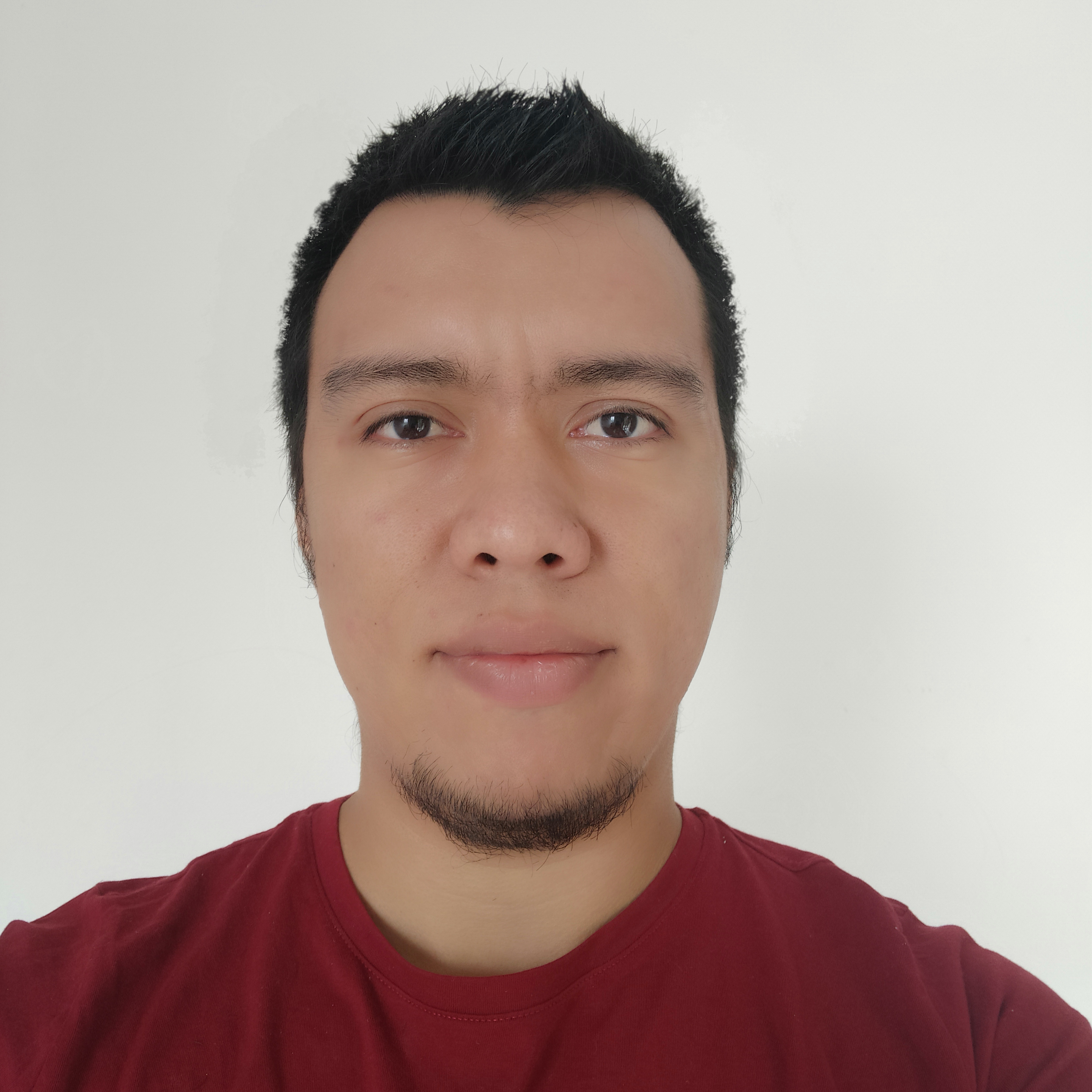
Thanks to TypeScript, it is possible to receive and return typed variables in functions. We already saw in a previous article how to define variables in TypeScript. In this post, we will go a little further and see their use in functions.
Number of arguments
First and before delving into types, let's see that in TypeScript even when a type is not defined for the parameters and a return value, there will be verification of the number of arguments passed to a function.
function sum(a, b)
{
return a + b;
}
sum(6);
The above code will throw the following error in the compiler for calling the function with only one parameter when two are required.
error TS2554: Expected 2 arguments, but got 1.
Function arguments
In TypeScript it is possible to declare a function with the type of its parameters. Consider the following example where we have defined a string type parameter.
function printName(userName: string)
{
return 'Hello ' + userName;
}
It is even possible to define a more complex parameter such as a structure in the function's signature. In the following example, the parameter must be an object that has the name and age attributes defined.
interface User {
name: string,
age: number
}
function printName(userName: User) {
return 'Hello ' + userName.name;
}
Return values
It is also possible to define the type that a function should return in TypeScript.
function printName(userName: string): string
{
return 'Hello ' + userName;
}
It is even possible to define a more complex return value such as a structure in the function's signature. In the following example, the return value must be an object that has the saludo and userName attributes defined.
function printName(userName: string): {
saludo: string,
userName: string
} {
return {
'saludo': 'Hello',
'userName': userName
};
}
The return value could even be an Interface (you can see more about interfaces in Interfaces in TypeScript).
interface User {
saludo: string,
userName: string
}
function printName(userName: string): User {
return {
'saludo': 'Hello',
'userName': userName
};
}
Functions as parameters
Functions in TypeScript can also be defined as parameters of other functions. These particular expressions in TypeScript are usually called function type expressions or function type expressions.
// a function that requires as a first parameter
// another function with a string argument
function greeter(fn: (a: string) => void) {
fn("Hello, World");
}
// a function that meets the parameter of the previous function
function printToConsole(s: string) {
console.log(s);
}
// passing a function as a parameter
greeter(printToConsole);
In the previous example, we can see how the function greeter
is defined, which requires a parameter named fn of type function. In turn, this function must comply with the signature (a: string) => void
.
Then, we define a function that complies with the signature of the parameter required in the initial function, and then this function is passed as a parameter when executing the greeter
function.
So far, you have almost all the tools to create and use your own TypeScript functions. If you want to go a little further, I recommend also seeing Call and Constructor Signatures in Typescript. See you next time.