Install Bootstrap 4.6 on Vue.js projects.
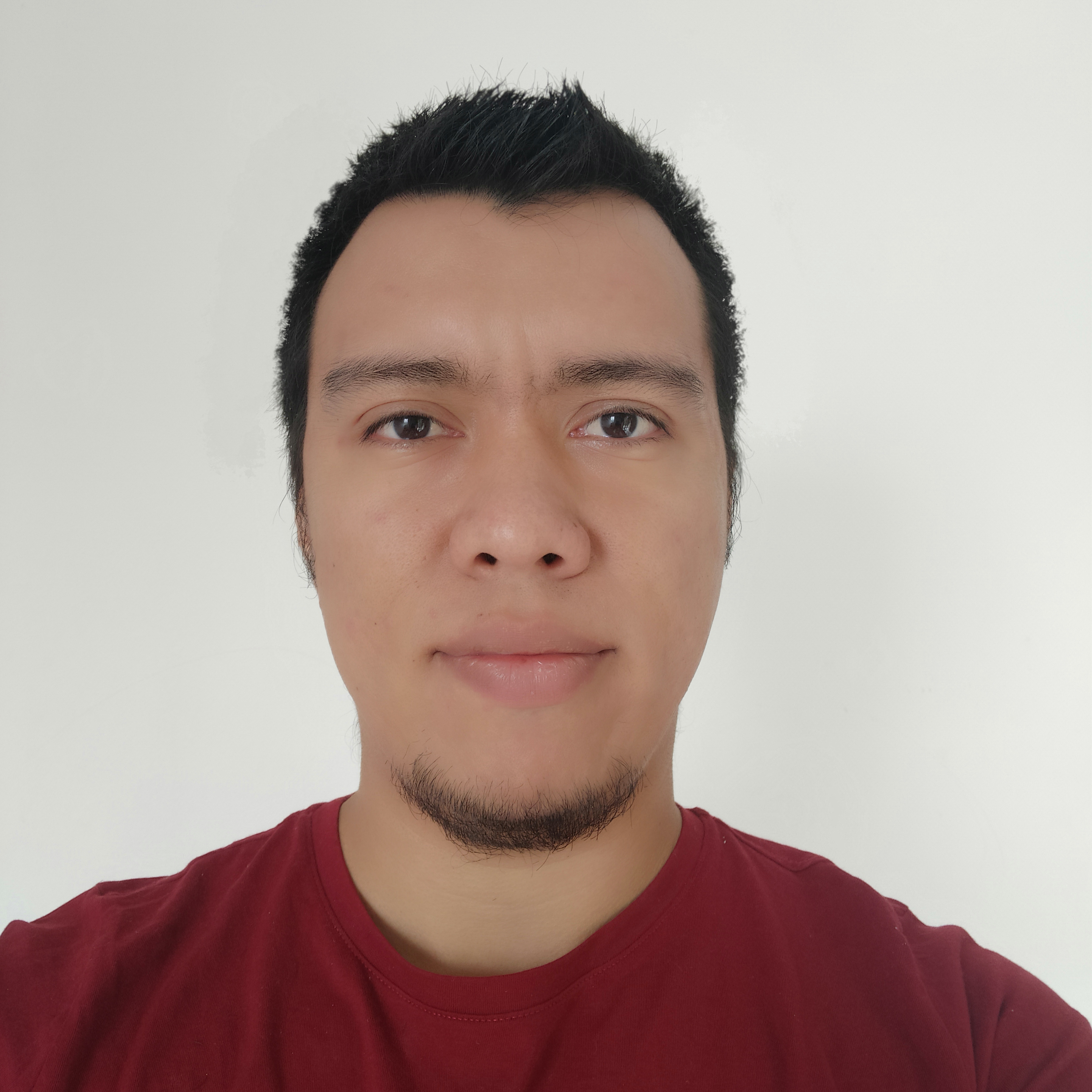
In a previous post we saw the structure or Application scaffolding in Vue.js. After this, you will most likely need to install a CSS library like bootstrap, Bulma, Semantic UI, etc. Today, we will see how to add Bootstrap 4.6 to our project in Vue.js.
The first thing you need to do is add Bootstrap.
npm i --save bootstrap@^4.6
If you just created your application in Vue.js you should not have to do anything else. Bootstrap 4.6 has dependencies like jQuery and Popper that will be automatically installed with bootstrap. To verify this, you can use the following commands.
user@server$ npm list --depth=1 | grep jquery
│ ├── jquery@3.6.0
user@server$ npm list --depth=1 | grep popper
│ └── popper.js@1.16.1
Or
user@server$ npm list jquery
my-project@0.1.0 /path/my-project
└─┬ bootstrap@4.6.0
└── jquery@3.6.0
user@server$ npm list popper.js
my-project@0.1.0 /path/my-project
└─┬ bootstrap@4.6.0
└── popper.js@1.16.1
After this, simply import bootstrap in the following way in the main.js file.
import "bootstrap";
import "bootstrap/dist/css/bootstrap.min.css";
If you just created your project in Vue, your main.js file will look very similar to the following.
import Vue from "vue";
import App from "./App.vue";
import "bootstrap";
import "bootstrap/dist/css/bootstrap.min.css";
Vue.config.productionTip = false;
new Vue({
render: (h) => h(App),
}).$mount("#app");
Once you have done this, you can start using bootstrap in your components. Until next time!.