The print function in Python
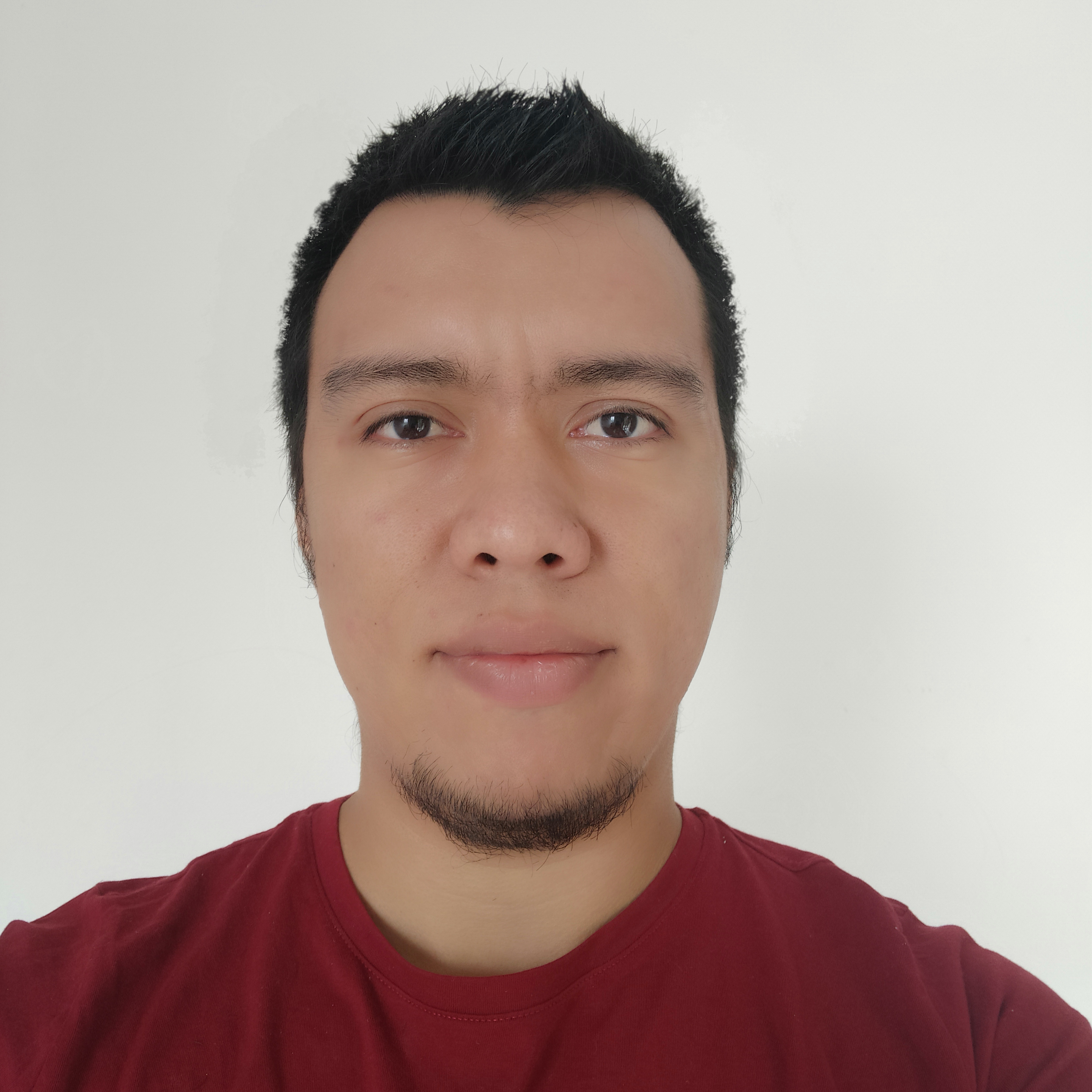
In general terms, the print function in Python allows you to print a string of text. However, there are several ways to solve the same problem with the print function as it is highly customizable. In this post, we will see everything about the print function.
Literals
Firstly, the print function can print a string literally without interpreting the string in any way.
print('Hello World')
You can use this syntax, for example, when you need to print quotation marks and you don't want them to be interpreted.
print('Hello "friend"')
Concatenation
The simplest way to concatenate in Python is with the +
operator, similar to how it is used in JavaScript.
print("Hello " + "world")
As you can see, we need to manually add the space character to separate the words. There is a much better way to do this by separating the strings in the function as if they were different arguments.
print("Hello", "world")
There is a third and less intuitive way, which involves using the print function multiple times for each string of text. As expected, Python adds a line break with each print function, so we need to override that behavior.
print("Hello", end=" ")
print("world")
Placeholder
We can also print the value of a variable using the format
function and the placeholder {}.
word = 'World'
print('Hello, {}' . format(word))
In Python 2 (legacy code), the way to do this was as follows:
word = 'World'
print('Hello, %s' % word)
F-strings
This approach is discouraged as it will be removed in later versions of Python. Starting from Python 3.6, it is possible to use F-strings to achieve the same result as the format function in the following way:
word = 'World'
print(f'Hello, {word}')
This latest syntax is much more descriptive in certain scenarios. Let's observe the following example.
n1 = 7
n2 = 9
print('{} is less than {}' . format(n1, n2))
When using F-strings, the message becomes much more explicit:
n1 = 7
n2 = 9
print(f'{n1} is less than {n2}')