What is ESLINT, what is it for and how to use it.
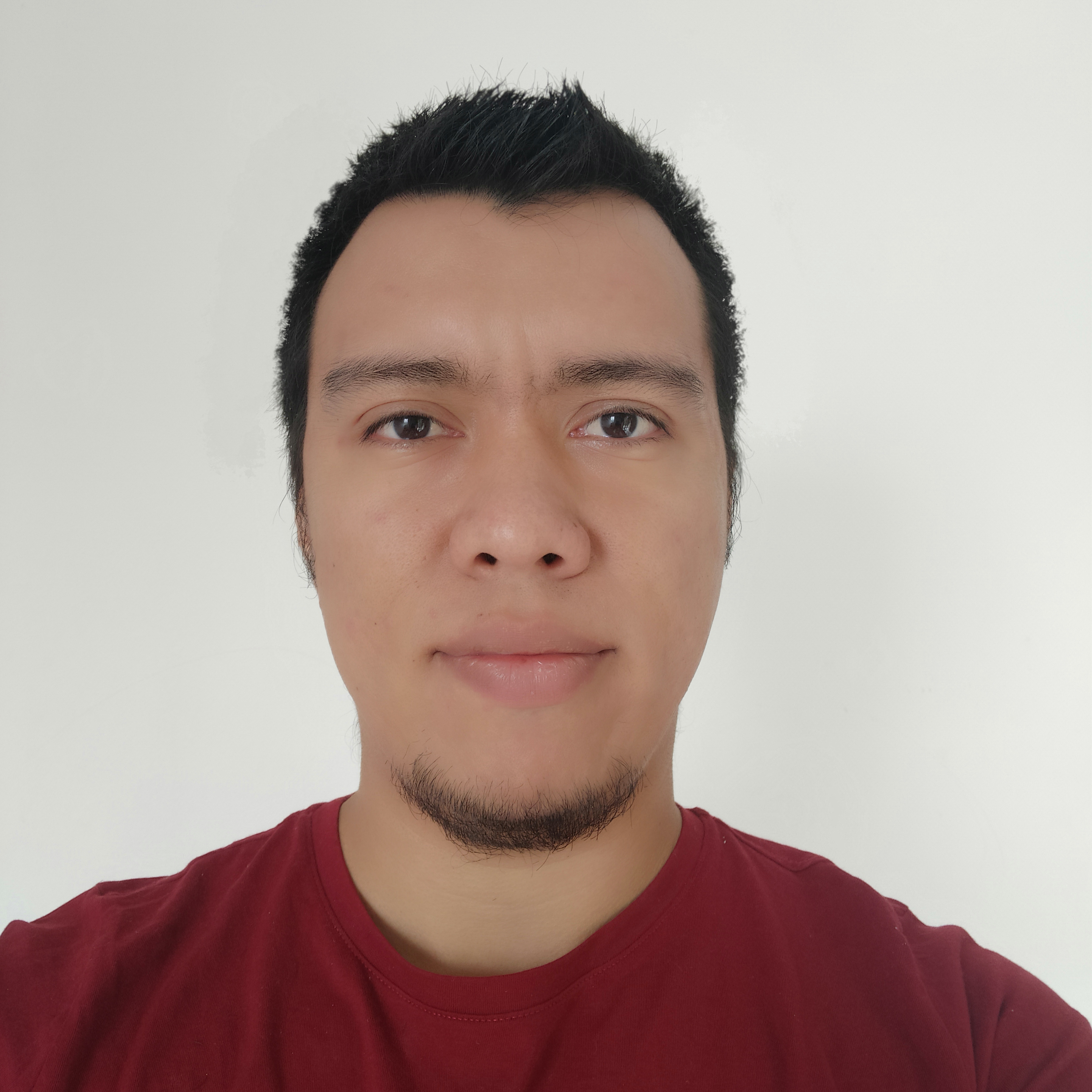
As we develop in JavaScript professionally, we encounter the problem of applying best practices across all our projects and the entire team. That's why there are tools like ESLINT for JavaScript which will help standardize development across our projects.
ESLINT is a linter for JavaScript. A linter is simply a tool that helps us comply with coding and styling best practices in a programming language. ESLINT is one of the most popular linters for JavaScript. But how do we know what the best practices are in a programming language? Well, for this there are presets, which are a set of established rules for best practices.
Installation
The first thing we need to do is install ESLINT using npm.
npm i --save-dev eslint
After this, we can use the following command to start the interactive configuration of eslint. This command requires that you already have a package.json file.
npm init @eslint/config
You should choose the answers based on the project you have. In my case, for testing eslint the following were the answers:
test » npm init @eslint/config
✔ How would you like to use ESLint? · style
✔ What type of modules does your project use? · none
✔ Which framework does your project use? · none
✔ Does your project use TypeScript? · No / Yes
✔ Where does your code run? · browser
✔ How would you like to define a style for your project? · guide
✔ Which style guide do you want to follow? · google
✔ What format do you want your config file to be in? · JSON
Checking peerDependencies of eslint-config-google@latest
The config that you've selected requires the following dependencies:
eslint-config-google@latest eslint@>=5.16.0
✔ Would you like to install them now with npm? · No / Yes
Installing eslint-config-google@latest, eslint@>=5.16.0
added 1 package, and audited 85 packages in 726ms
13 packages are looking for funding
run `npm fund` for details
found 0 vulnerabilities
Successfully created .eslintrc.json
As you can see, I used the google preset, which currently works with the latest version of ESLint (Google Standard).
By using this preset, you'll see that a file called .eslintrc.json is created in the root folder of your project with the following content:
{
"env": {
"browser": true,
"es2021": true
},
"extends": [
"google"
],
"parserOptions": {
"ecmaVersion": "latest"
},
"rules": {
}
}
If you use a code editor like Visual Studio Code and have the ESLint plugin installed, you'll see that errors are automatically marked.
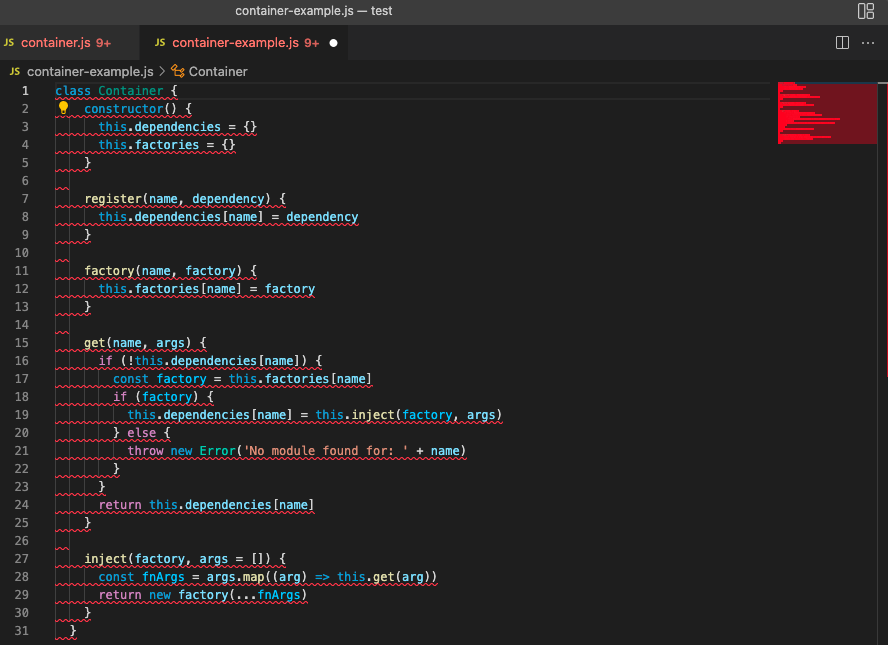
Configuration
You can add a pair of scripts to facilitate the use of the library. I personally use the following:
"scripts": {
"lint-check": "npx eslint index.js ./src/**/*.js --fix-dry-run",
"lint": "npx eslint index.js ./src/**/*.js --fix",
}
Usage
To see eslint in action, we're going to create the man.js file with the following content.
window.onload = function() {
document.querySelector("body").innerHTML = '<h1>Hello World!</h1>'
}
const upper =function( text) {
return text.toUpperCase();
}
Once we've done this, we can run the eslint command to verify the main.js file.
npx eslint main.js --fix-dry-run
We'll get an output like the following:
/path/dariorivera/www/test/foo.js
2:1 error Expected indentation of 2 spaces but found 3 indent
2:27 error Strings must use singlequote quotes
2:70 error Missing semicolon semi
3:2 error Missing semicolon semi
5:7 error 'upper' is assigned a value but never used no-unused-vars
6:1 error Expected indentation of 2 spaces but found 0 indent
7:2 error Newline required at end of file but not found eol-last
7:2 error Missing semicolon semi
✖ 8 problems (8 errors, 0 warnings)
7 errors and 0 warnings potentially fixable with the `--fix` option.
To have eslint make the necessary corrections to the files, we need to change the --fix-dry-run command to --fix:
npx eslint main.js --fix
Our file will look like this:
window.onload = function() {
document.querySelector('body').innerHTML = '<h1>Hello World!</h1>';
};
const upper =function( text) {
return text.toUpperCase();
};
Still, if you observe the output of the command there's still one error left.
/path/dariorivera/www/test/foo.js
5:7 error 'upper' is assigned a value but never used no-unused-vars
✖ 1 problem (1 error, 0 warnings)
Which is completely logical since that variable isn't used.
In this article, you learned what ESLINT is and how to use it through commands in your project. However, ESLINT can also be used integrated with Webpack in a much more standard way. I invite you to check out the following links to carry this out. Until next time!
- Integrate ESLINT with Webpack
- Integrate ESLINT with Vue.js