Routes in Laravel
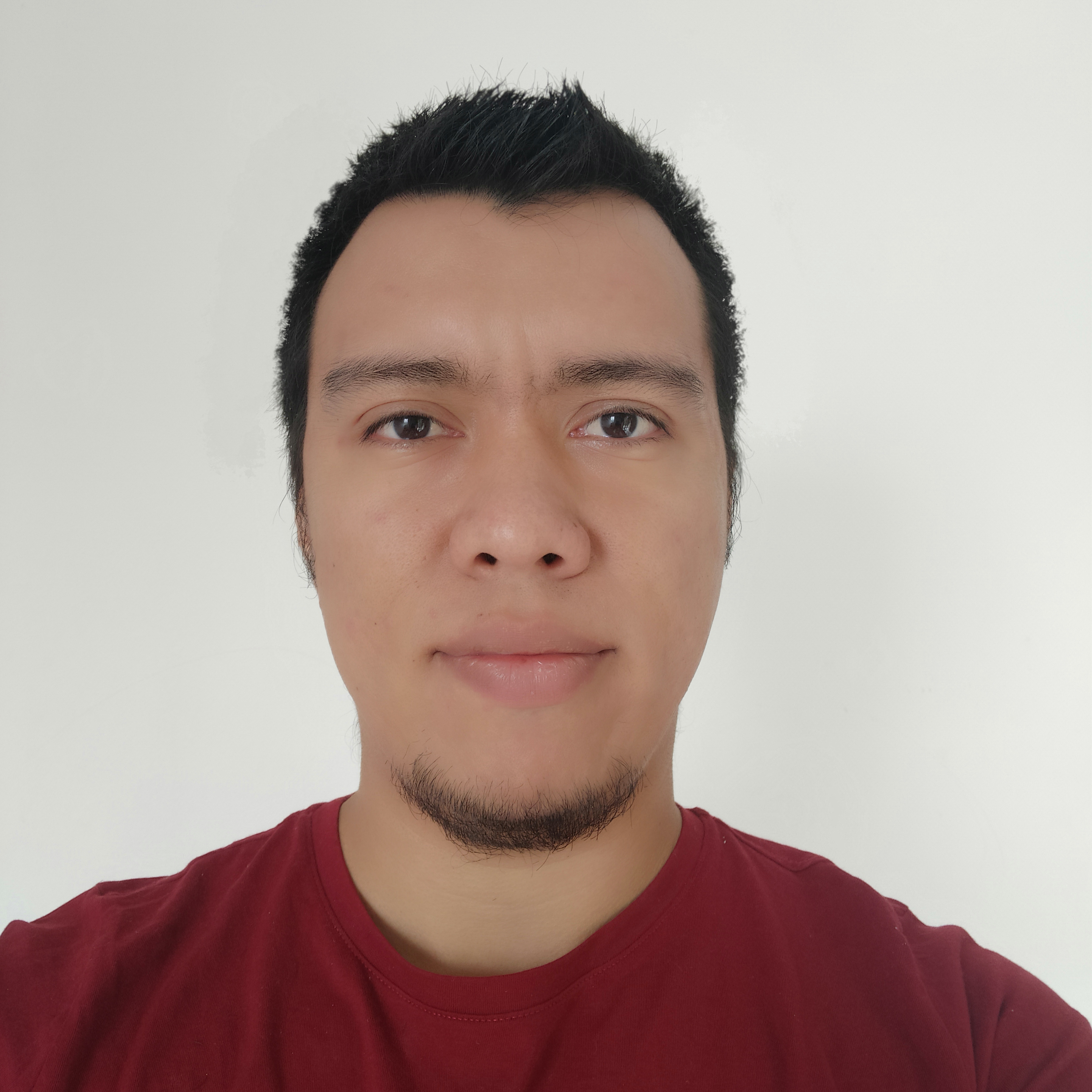
Routes in Laravel are defined in the files that you can find in the routes folder. From these files, we will focus mainly on two: web.php and api.php. These two files define the routes for the web application and for the API (almost always RESTful). The behavior of the router can be said to be the same when we are defining any type of route, that being said, we will see some generalities before observing how it is used on one side or the other.
The definition of a route has many variations, let's see what I believe is the simplest definition.
Route::get('/user', 'UserController@index');
This simple line of code tells Laravel that when making a GET request on the route /user, execute the index
method of the UserController
controller. Another variation more general than the previous one accepts a callback as the second parameter as can be seen in the following examples.
Route::get($uri, $callback);
Route::post($uri, $callback);
Route::put($uri, $callback);
Route::patch($uri, $callback);
Route::delete($uri, $callback);
Route::options($uri, $callback);
For each HTTP verb, Laravel implements a static method with the same name that only accepts requests for the defined verb. In this way, a particular example could be the following:
Route::get('/', function () {
return view('welcome');
});
The previous definition comes by default in a clean installation of Laravel. The callback passed as the second parameter of the get
function tells Laravel to return or display the welcome view without the need to go through a controller. This view corresponds to the welcome.blade.php file in the resources/view folder. However, there is a much shorter way if you just want to return a view. The following example is equivalent to the previous one.
Route::view('/', 'welcome');
In addition, if you need to pass any parameter or message to the view (see how in our post MVC Pattern in Laravel), you could use an additional parameter for this:
Route::view('/welcome', 'welcome', ['message' => 'Hello world!']);
If a method of your controller or a callback needs to go through more than one HTTP verb, there is no need to repeat the definition of the route, just use the following variant:
Route::match(['get', 'post'], '/', function () {
// match get and post
});
If the request is open to any HTTP verb, just use the any
method.
Route::any('foo', function () {
// match any verb
});
To sum up, remember that routes to access with URLs in the browser are defined, as mentioned, in the web.php file. Routes to access an API are defined in the api.php file. Any route defined in this file will by default have the prefix /api followed by the route name. See you soon!.