Setup Gmail API to send e-mails
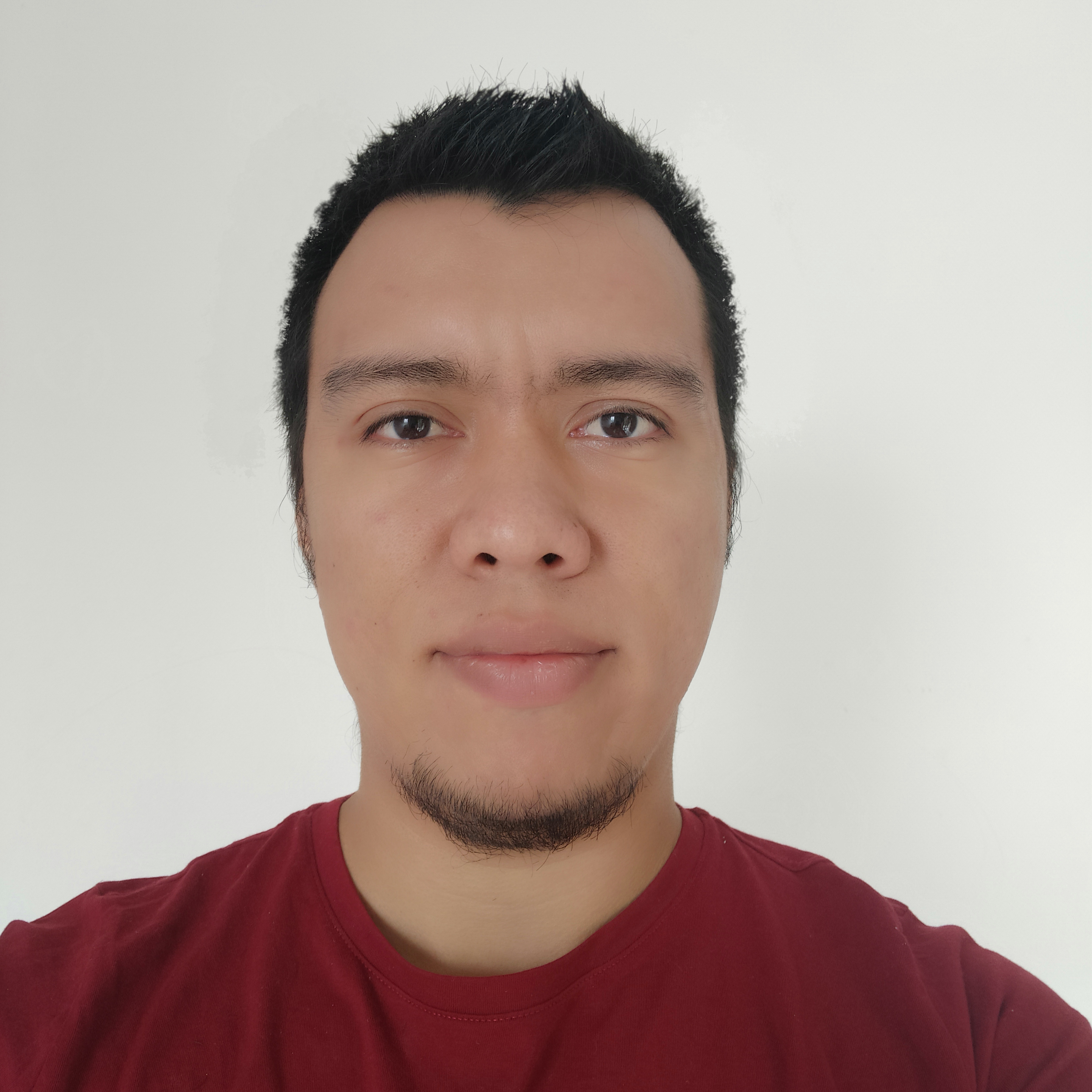
The Gmail API is an application programming interface provided by Google that allows developers to interact with Gmail, Google's email service. It provides a set of methods and resources to access, manage, and manipulate a user's Gmail account programmatically. The API enables developers to create, read, update, and delete email messages, labels, threads, and drafts.
Use cases
Email Client Integration: Developers can utilize the Gmail API to build custom email clients that offer unique features and functionalities on top of Gmail. This allows users to access their Gmail accounts, send and receive emails, manage labels and threads, and perform advanced searches from within the custom client.
Workflow Automation: By leveraging the Gmail API, developers can automate email-based workflows. For example, they can create applications that automatically process incoming emails, extract relevant information, and trigger actions based on specific criteria, such as forwarding emails to different recipients, saving attachments to cloud storage, or generating automatic responses.
Email Analytics and Monitoring: The Gmail API enables developers to extract email data, such as message metadata, thread information, and email content. This data can be used to build analytics tools that provide insights into email usage patterns, perform sentiment analysis on email conversations, or monitor email communication for compliance purposes.
Requirements to consume the API
Before starting to consume the API we will need the following:
- A project in the Google Cloud Console
- Enable Gmail API
- Create API keys
Create a project in the Google Cloud Console
To get started, the initial step involves creating a Google Cloud project. If you happen to already possess an existing project, feel free to bypass this particular step. To determine whether you already have an active project, follow these simple instructions:
- Go to the Google Cloud Console.
- Locate the top navigation bar, and from there, select the current project.
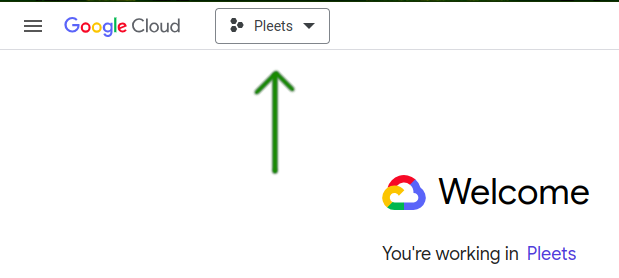
- Click "Create project" or "New Project".
- Enter a name for the project and click "Create". Optionally you can select the organisation in which you want to create the new project.
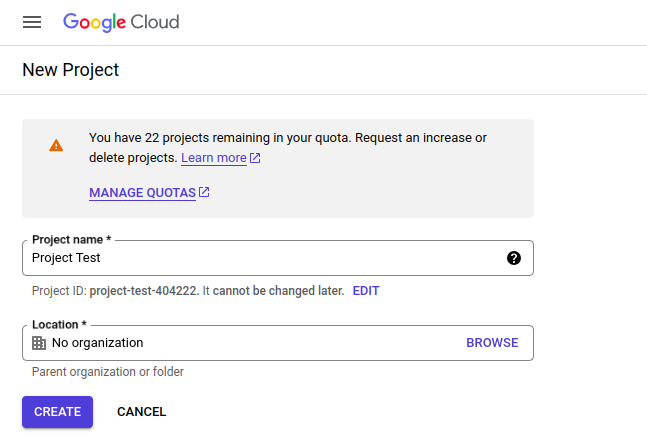
Enable Gmail API
The first thing you need to do is go to the Official API implementation documentation. You must click on the use the setup tool link to sign up in Google Cloud if you haven't done it before. In that case, you'll see a modal confirmation to accept the terms of service. Look at the project on top, you can change it if you're in the wrong one.
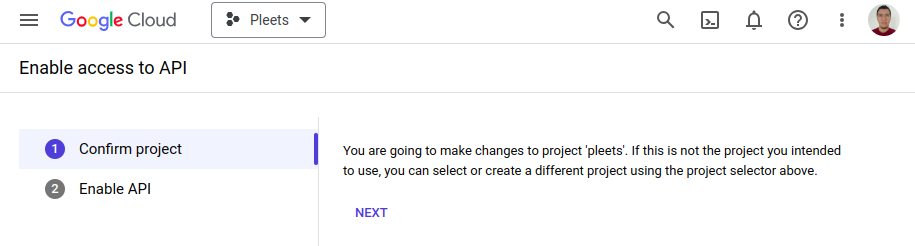
Next, you need to click "Enable" to make the API services available in your Google Cloud Console..
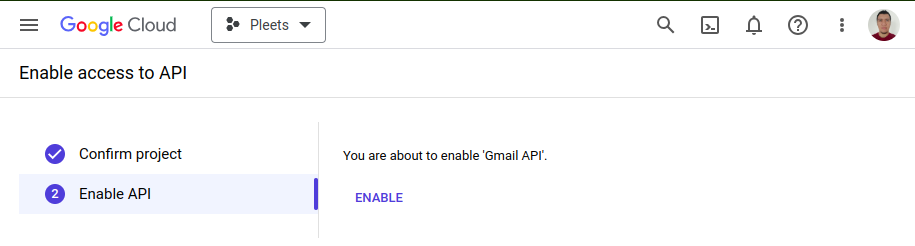
Create API Keys
Once that's done, you could go to the left bar to API & Services > Credentials.
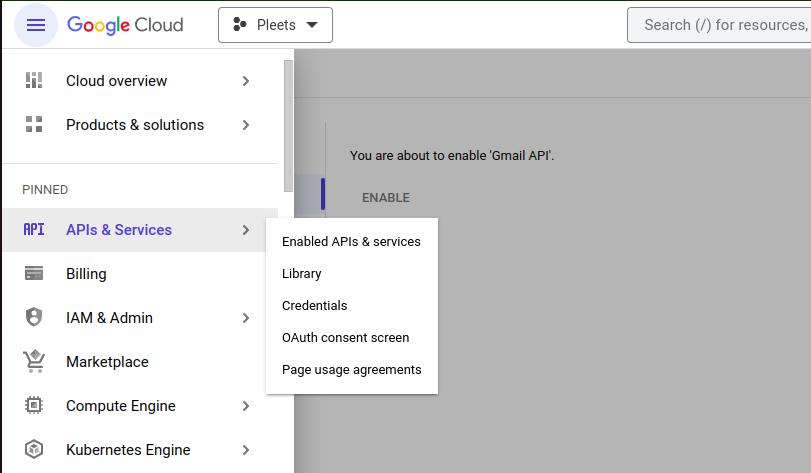
Click the button + Create Credentials and select API Key.
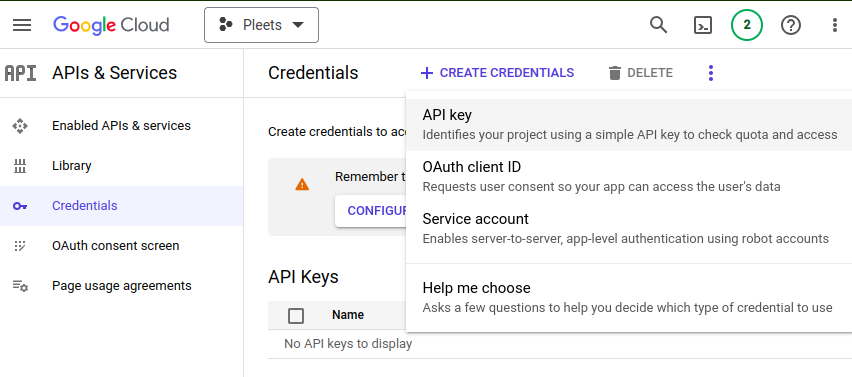
You'll see a confirmation modal.
API key Restricting access
Once a new key is created you'll see a confirmation alert like the following.
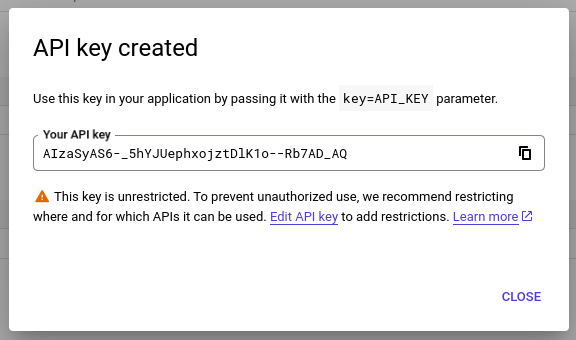
Click "Edit API Key" and restrict the access as you needed. You can restrict access to allow certain IPS or websites to use the API key.
Consume the API
Once you have all the necessary configuration and tools, consuming the API is relatively straightforward. Depending on the programming language you are using, you will need to use a specific library. In the following example, we use Python to send a simple Email.
import os
from google.auth.transport.requests import Request
from google.oauth2.service_account import Credentials
from googleapiclient.discovery import build
# Gmail API key
api_key = 'TU_API_KEY_DE_GMAIL'
# Gmail API scope
SCOPES = ['https://www.googleapis.com/auth/gmail.compose']
def create_gmail_service():
# Crete credentials using the API key
creds = Credentials.from_authorized_user_info(api_key, scopes=SCOPES)
# Update credentials if was expired
if creds and creds.expired and creds.refresh_token:
creds.refresh(Request())
# Create an instance of the Gmail service
service = build('gmail', 'v1', credentials=creds)
return service
def send_email(sender, to, subject, message):
service = create_gmail_service()
email_message = f"From: {sender}\nTo: {to}\nSubject: {subject}\n\n{message}"
# send the email using Google Gmail API
message = (service.users().messages().send(
userId='me',
body={'raw': base64.urlsafe_b64encode(email_message.encode()).decode()}
).execute())
print("Email sent correctly.")
# Usage example
sender = 'youremail@gmail.com'
recipient = 'receivar@example.com'
subject = 'Hello from GMAIL API'
message = '¡Hello!\n\nThis email was sent using the Google Gmail API.'
send_email(sender, recipient, subject, message)
En which:
TU_API_KEY_DE_GMAIL
Is the API key obtained in previous steps.