Java Decision Statements (switch)
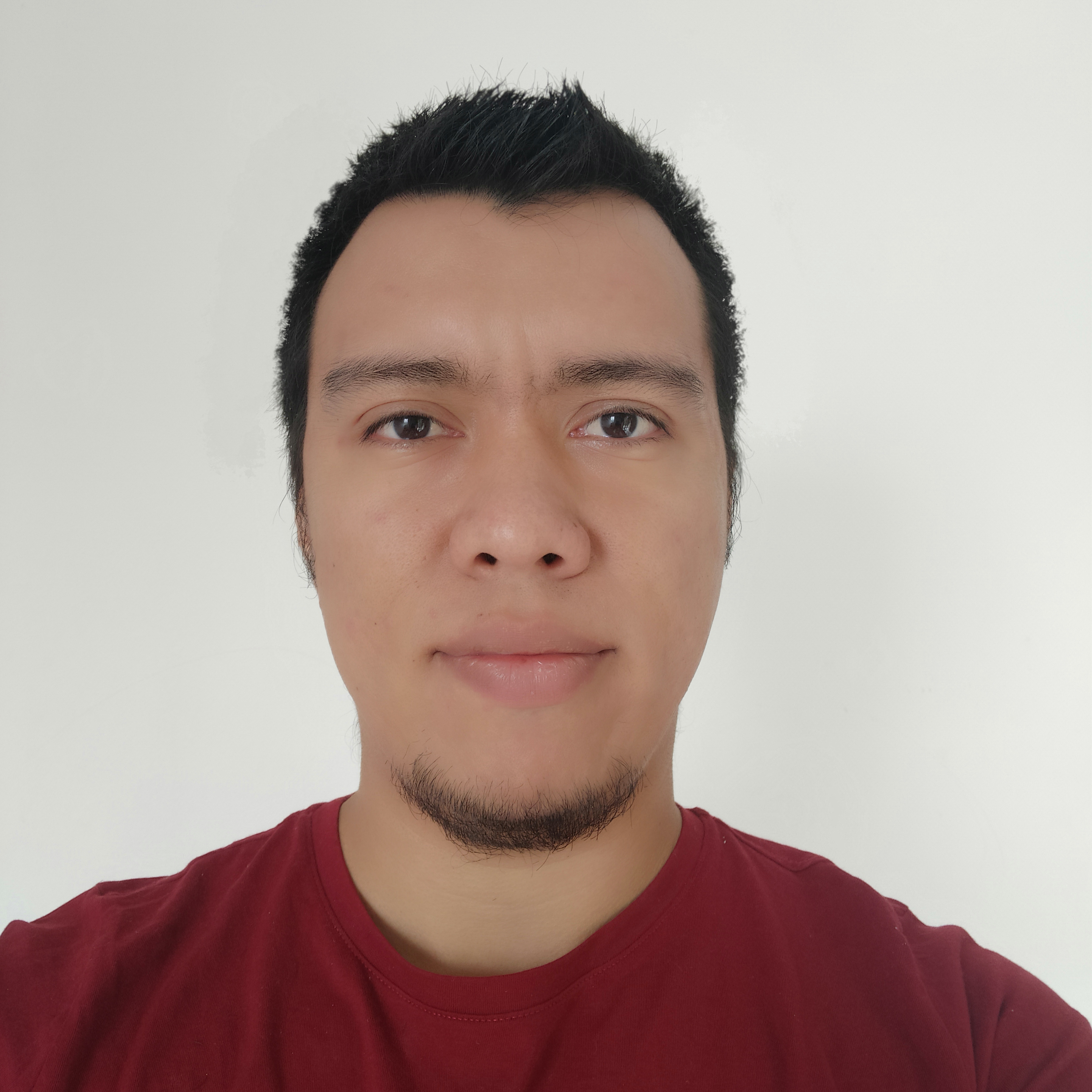
In our previous post we saw the Decision Statements in Java (if-then, if-then-else), today we will complete this topic with the Switch statement. Remember that control statements allow us to execute specific blocks of code based on a decision. However, there is a substantial difference between the switch statement and the other decision statements that we will see below.
Mutually exclusive conditions
Just like with the if-then
, if-then-else
statements, we can build a series of conditions that will allow only one block of code to be executed. Let's see the following example.
public class Test
{
public static void main(String[] args) {
int day = 3;
String dayName;
switch (day) {
case 1:
dayName = "Monday";
break;
case 2:
dayName = "Tuesday";
break;
case 3:
dayName = "Wednesday";
break;
case 4:
dayName = "Thursday";
break;
case 5:
dayName = "Friday";
break;
case 6:
dayName = "Satudary";
break;
case 7:
dayName = "Sunday";
break;
default:
dayName = "Invalid day";
}
System.out.println(dayName);
}
}
When running this code, the result obtained is
Wednesday
This happens because the variable day is equal to 3 and meets the condition case 3. In addition, each case block ends with the break
statement, which prevents subsequent blocks from being executed. Note also that the last block is default
. This block is necessary because if it is not placed, the program will not compile. If none of the conditions are met, the variable will be initialized to the default value, which in this case is "Invalid day".
Non-exclusive conditions
Unlike the if-then
, if-then-else
statements, the switch
statement allows the execution flow to follow through several paths and not just one. Let's see the following example.
public class Test
{
public static void main(String[] args) {
int years = 4;
int bonus = 0;
switch (years) {
case 5:
bonus += 1000;
case 4:
bonus += 0;
case 3:
bonus += 500;
case 2:
bonus += 0;
case 1:
bonus += 100;
default:
break;
}
System.out.println("Accumulated Bonus: " + bonus);
}
}
When running this code, the result obtained is
Accumulated Bonus: 600
The goal of this program is to calculate the accumulated bonus that a person working in a company would have, in which the employee receives $100 in the first year, $500 in the third year, and $1000 in the fifth year. As you can see, a person who has been working for four years will have an accumulated bonus of $600, just as shown in the program's output. This is because, as you may have noticed, the condition enters the case 4 statement which adds 0 USD to the bonus variable. Since this block does not have a break
, the program will continue executing the other blocks regardless of the value of the expression in the case
labels. That is, it will have added to the bonus variable 0 + 500 + 0 + 100. Note that the switch block will finish its execution on the first break
statement that it finds.
Multiple case labels
Case statements with the same block of code can be grouped. Let's suppose now that the government will give an incentive to mothers for their children under 6 years old. For a child 1 or 2 years old, it will give an incentive of 100 USD, for a 3-year-old child it will give 500, for a 4-year-old it will give 1000 and for one of 5 it will give 2000. Given this, we can write the following program.
public class Test
{
public static void main(String[] args) {
int age = 1;
int bonus = 0;
switch (age) {
case 1:
case 2:
bonus = 100;
break;
case 3:
bonus = 500;
break;
case 4:
bonus = 1000;
break;
case 5:
bonus = 2000;
break;
default:
break;
}
System.out.println("Incentive: " + bonus);
}
}
Note that the change is as subtle as it is elegant. Since the first and second years receive the same amount per child, there is no need to place the same condition in the case 2 block. The output of the program will be the following, taking the variable age as the age of the child.
Incentive: 100
Finally, note that a switch statement can evaluate an integer, an enumerated value, or a string. See you soon!