Functions in Go
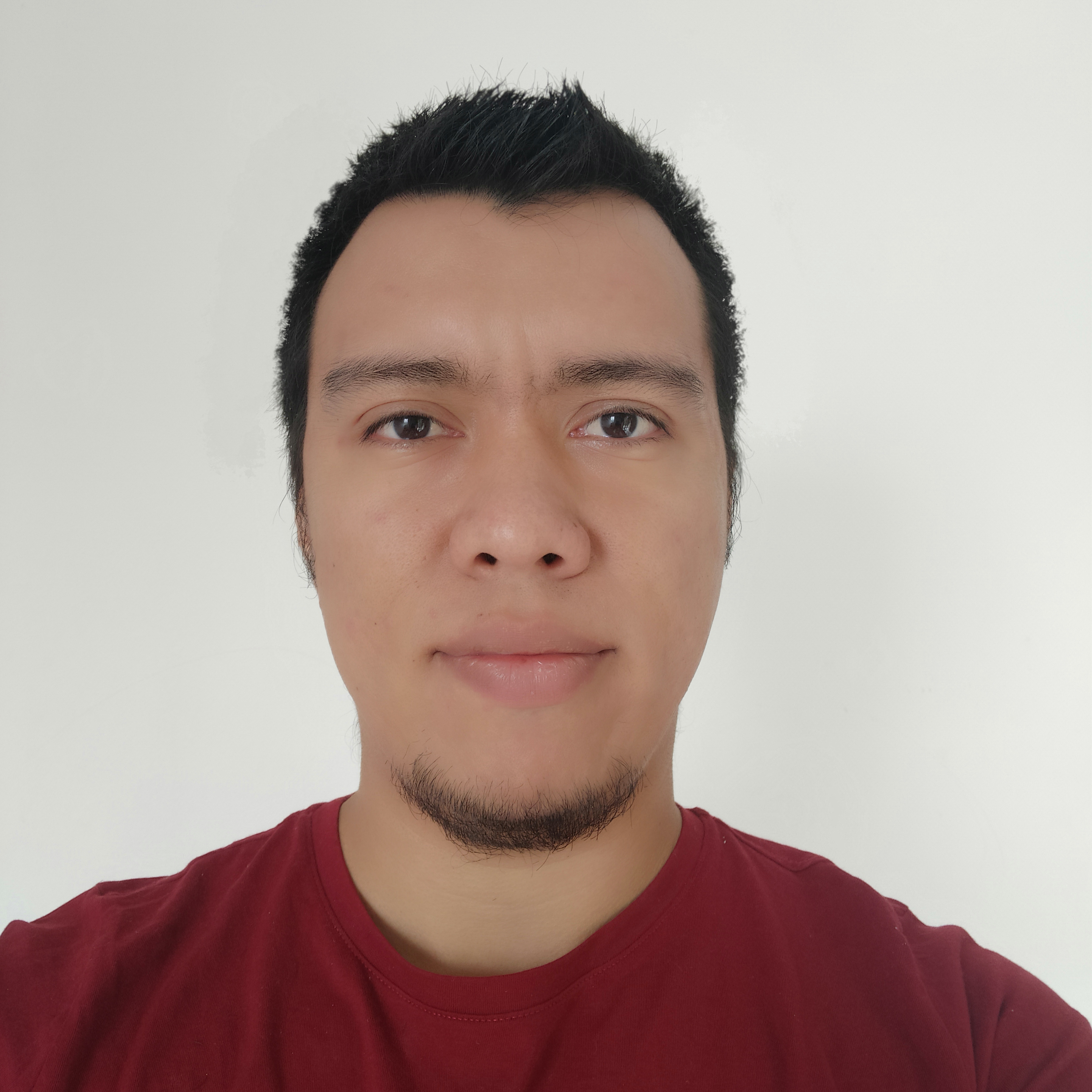
In a previous post we have seen in a general way the structure of a program in Go, today we will see a very important concept in Go which are functions. For this, let's observe the following program:
Function Definition
For the definition of functions in Go let's take as a base the following program.
package main
import "fmt"
func add(x int, y int) int {
return x + y
}
func sub(x, y int) int {
return x - y
}
func main() {
fmt.Println("42 + 13 =", add(42, 13))
fmt.Println("42 - 13 =", sub(42, 13))
}
And let's see that:
( 1 ) The reserved word func
defines the declaration of a function.
( 2 ) The type of a function parameter goes after the name of said parameter.
( 3 ) When two or more consecutive parameters share the same type, the type of all but the last one can be omitted.
Multiple Return Values
A function in Go can return more than one value simultaneously.
func swap(x, y string) (string, string) {
return y, x
}
func main() {
a, b := swap("hello", "world")
fmt.Println(a, b)
}
Note that what was previously a return value type is now a pair of return values enclosed in parentheses (string, string
). When using a function that returns more than one value simultaneously, it is necessary to indicate each variable in the assignment of said return values (a, b :=
).
Named Return Values
Return values can be named just like function parameters.
func split(sum int) (x, y int) {
x = sum * 4 / 9
y = sum - x
return
}
In this case, it is enough to make an assignment on the names of the return variables and use a "naked return" to return the values. Like return values for functions that return more than one value, named return values must be enclosed in parentheses regardless of whether a single value or multiple values are returned.
In an upcoming post we will see the data types in Go. Until next time.