Guide to Sass for Beginners
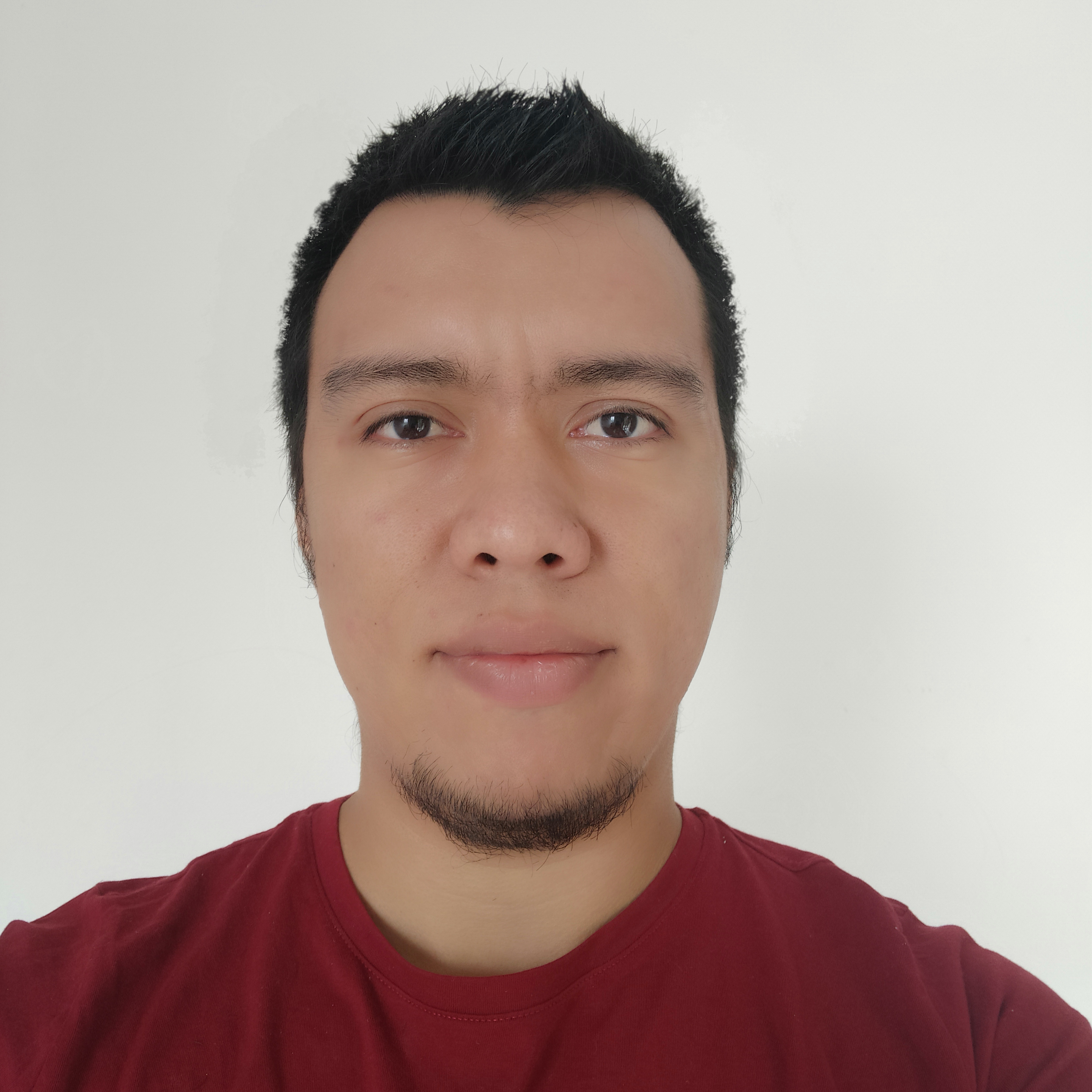
Sass is a stylesheet language that can be compiled to CSS. This means it can't be used directly in the browser but by compilation tools it can be rewritten to be browser compatible.
The biggest advantage of Sass is that you can reuse your CSS code and develop styles much faster and consistently than with pure CSS. In this quick guide you will learn how to install and use Sass so that you can start using it in your web projects.
Installation
There are several ways to install Sass. However, in this guide we'll see how to add it to a project that handles its dependencies with npm. You probably already have a project running in Laravel or any other web development framework. It shouldn't be difficult after following these steps to add it to your specific project.
The first thing we're going to do is to create an initial project in npm with the following command.
npm init -y
This command will create the package.json file where the configuration of our project dependencies will be. The next step will be to install Sass as a development dependency.
npm i -D sass
Sass Execution
To execute Sass we're going to create two commands in the scripts area of our package.json file.
"scripts": {
"build": "sass src/styles.scss css/styles.css",
"watch": "sass src/styles.scss css/styles.css --watch"
}
The first command takes what's in the src/styles.scss file, applies the processing of the file and finally generates the css/styles.css file. The second command does exactly the same thing, but the command will remain running in the terminal so that as we modify our styles, the output is generated automatically.
Sass Syntax
Let's see one by one some of the most popular Sass features.
Sass markup
You can nest your styles just as you would in HTML.
Syntax
body {
background: white;
h1 {
color: black;
}
}
CSS
body {
background: white;
}
body h1 {
color: black;
}
Variables
With the use of variables you can use the same value throughout the entire CSS code and easily change it when required.
Syntax
$base-color: white;
.btn-#{$base-color} {
background-color: $base-color;
}
.base-background {
background: url("/icons/bg/#{$base-color}");
}
CSS
.btn-white {
background-color: white;
}
.base-background {
background: url("/icons/bg/white");
}
Flow control
With Sass, you can also have flow control just as you would in most programming languages.
@if
Syntax
$base-color: white;
@if $base-color == white {
.base-contrast {
background-color: black;
}
}
CSS
.base-contrast {
background-color: black;
}
@each
Syntax
$dir: assets/icons;
@each $icon in (foo, bar, baz) {
.icon-#{$icon} {
background: url('#{$dir}/#{$icon}.png');
}
}
CSS
.icon-foo {
background: url('assets/icons/foo.png');
}
.icon-bar {
background: url('assets/icons/bar.png');
}
.icon-baz {
background: url('assets/icons/baz.png');
}
@for
Syntax
@for $count from 1 to 5 {
@if $count > 2 {
.col-#{$count} {
width: #{$count} + 0%;
}
}
}
CSS
.col-3 {
width: 30%;
}
.col-4 {
width: 50%;
}
Using through instead of to also includes the final number.
@mixin and @include
With @mixin
we can define styles that can be added with @include
elsewhere in the code.
Syntax
@mixin heading-text {
color: #242424;
font-size: 4em;
}
h1, h2, h3 {
@include heading-text;
}
CSS
h1, h2, h3 {
color: #242424;
font-size: 4em;
}
With @mixin
we can also pass parameters just as if it were a function.
Syntax
@mixin heading-text($color: #242424, $font-size: 4em) {
color: $color;
font-size: $font-size;
}
h1, h2, h3 {
@include heading-text(#111111, 6em);
}
CSS
h1, h2, h3 {
color: #111111;
font-size: 6em;
}