Decision statements in Java (if-then, if-then-else)
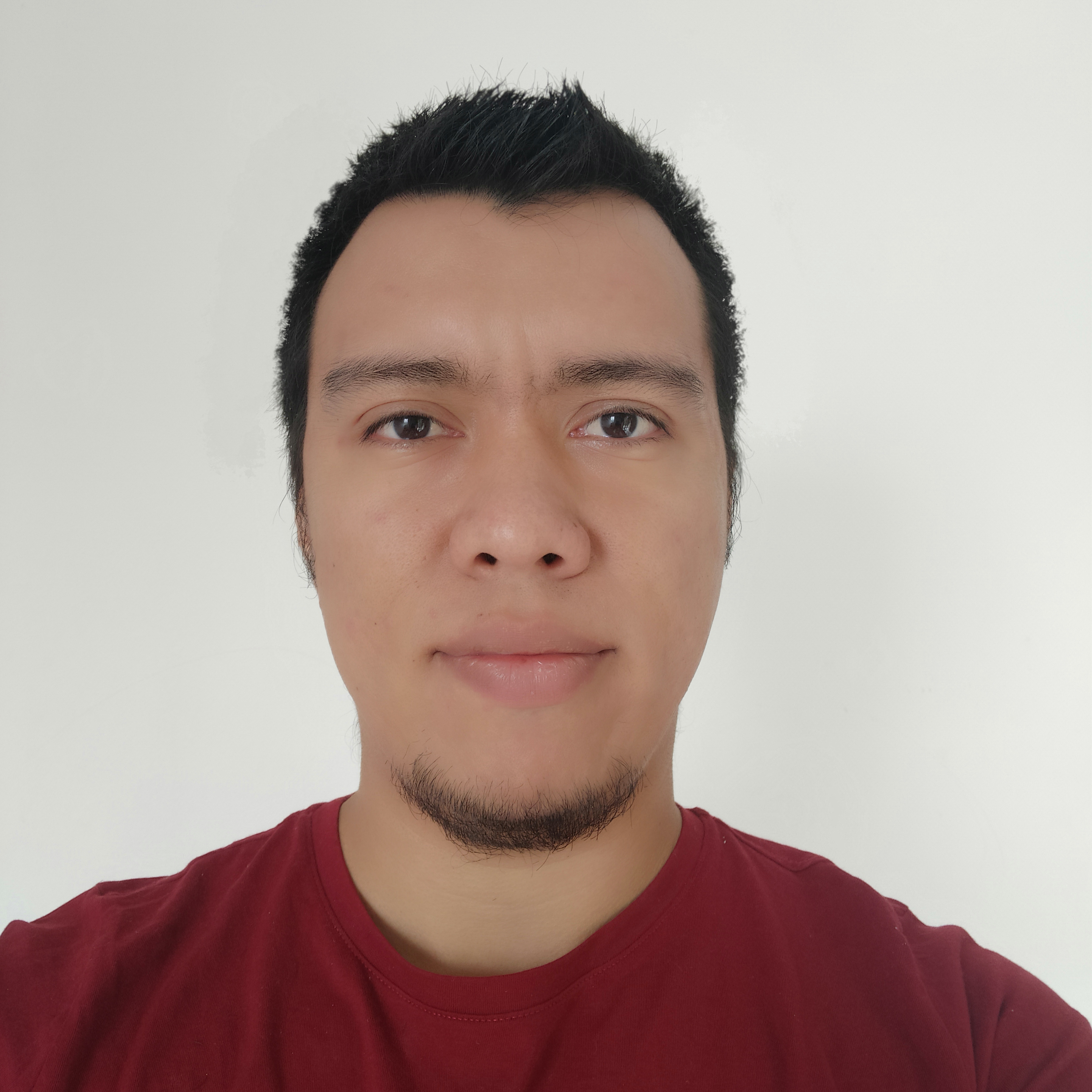
Control flow statements allow us to execute specific code blocks based on a decision. Remember that the general flow of a program is from top to bottom, that is, it is executed from the first line to the last. In this post, we will see how to code the if-then and if-then-else decision statements in Java.
If-Then Statement
This statement allows to execute a code block if the evaluation of a condition results in true
. Let's see the following example.
public class Test
{
public static void main(String[] args) {
int number = 10;
if (number > 7) {
System.out.println("The number is greater than 7");
}
}
}
When running this code, we get the following.
The number is greater than 7
This means that the evaluation performed resulted in true
since 10 > 7
.
If-Then-Else Statement
This statement adds an alternative path when the condition is evaluated as false
. Let's alter a little bit the previous example and see the following.
public class Test
{
public static void main(String[] args) {
int number = 5;
if (number > 7) {
System.out.println("The number is greater than 7");
} else {
System.out.println("The number is lower than 7");
}
}
}
When running this code, we get the following.
The number is lower than 7
This means that the evaluation performed resulted in false
since 5 < 7
. At this point you might be thinking, what if we have more than two simple conditions?. Let's see the following example reformed from the previous one.
public class Test
{
public static void main(String[] args) {
int number = 7;
if (number > 10) {
System.out.println("The number is greater than 10");
} else if (number > 5) {
System.out.println("The number is greater than 5");
} else {
System.out.println("The number is lower than 5");
}
}
}
When running this code, we get the following.
The number is greater than 5
This is because the first condition was false
since 7 < 10
. Given this, the second condition is evaluated and results in true
since 7 > 5
. The last block is skipped since the execution entered through one of the preceding conditions.
Complex Conditions
Decision statements can evaluate a decision composed of a complex expression. Let's see the following example.
public class Test
{
public static void main(String[] args) {
int number = 7;
if (number >= 5 && number <= 10) {
System.out.println("The number is between 5 and 10");
} else {
System.out.println("The number is not between 5 and 10");
}
}
}
When running this code, we get the following.
The number is between 5 than 10
The &&
operator indicates that both conditions must be met. Additionally, there are alternative ways to evaluate a condition, such as the ternary operator. Do you remember the first example of the if-then-else statement?. Well, it can also be written in the following way.
public class Test
{
public static void main(String[] args) {
int number = 5;
String comparision = (number > 7) ? "greater" : "lower";
System.out.println("The number is " + comparision + " than 7");
}
}
When running this code, we get the following.
The number is lower than 7
It behaves exactly the same as the first example. The difference is that the conditional is executed to assign a String
to the variable comparison. In this case, the block that is executed is "lower" and not "greater". When the condition is evaluated as true
, the block after the question mark (?) is executed, when it is evaluated as false
, the block after the colon ( : ) is executed.