Install and configure TypeScript.
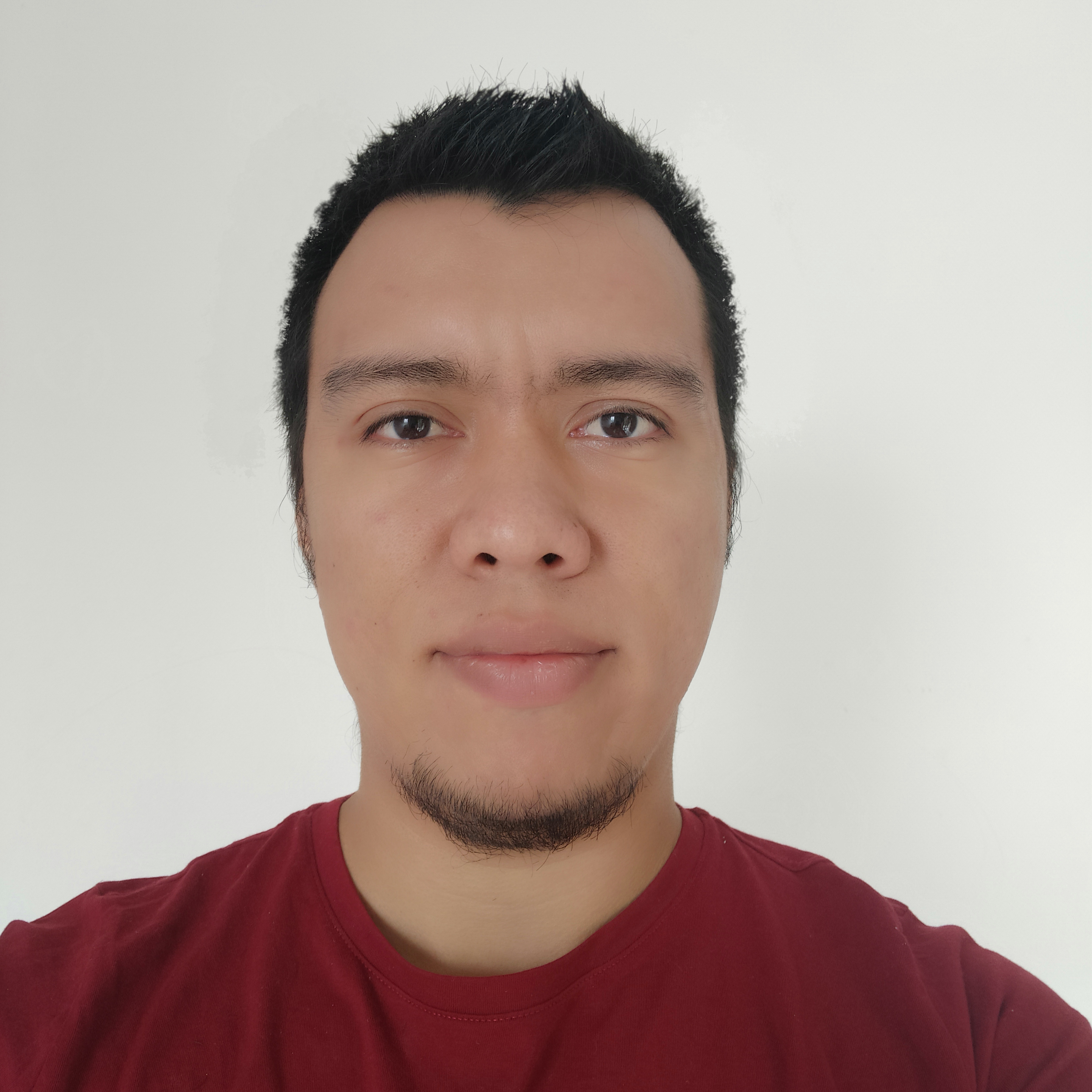
TypeScript is a strongly typed programming language based on JavaScript. In fact, it is a superset of JavaScript. In this post we will see how to install it and how to configure it in any existing or new project.
Installation
To install typescript you just need to execute the following command. This will allow you to use typescript in any directory.
npm install -g typescript
Once installed, you can see the installed version with the following command:
server@user$ tsc --version
Version 4.8.2
Configuration
To configure typescript, create a file in the root folder of the project called tsconfig.json
with the following scaffolding:
{
"compilerOptions": {
...
}
}
You could even create the configuration file with an empty object {}
and TypeScript will take all default values.
Target
This option tells the compiler which version of ECMAScript will be generated as resulting code, or in other words, which version it is compatible with. If no version value is specified, ES3 will be taken as default. Example:
{
"compilerOptions": {
"target": "es5"
}
}
If you are using some compatibility tool like Babel, you could set the value esnext
which basically removes all metadata types and passes the rest of the Javascript code untouched.
{
"compilerOptions": {
"target": "esnext"
}
}
outDir
This option tells typescript where to place the transpiled JavaScript code. If no value is specified, the current directory will be taken as default.
{
"compilerOptions": {
"outDir": "dist"
}
}
strict
This option allows activating strict mode in typescript.
{
"compilerOptions": {
"strict": true
}
}
Sometimes having strict
enabled can be a headache since it is the most restrictive mode of TypeScript. That is why this option is more of a set of options, so we can choose to deactivate it and activate other individual restrictions such as noImplicitAny
or strictNullChecks
. You can see a little more in-depth about this option in Compilation of Programs in TypeScript.
Transpilation
Once these configurations have been made, it's time to test that everything has been set up correctly. For this, we will create a file called User.ts with the following content:
class User
{
constructor(name)
{
this.name = name
}
}
In another terminal window you will run the following command in the project directory or where you created the file.
tsc -w
This creates a live mode that as you make changes to the code you will see the transpilation with the file description. If everything went well, you will get an output similar to the following:
[8:31:56 PM] File change detected. Starting incremental compilation...
User.ts:5:14 - error TS2339: Property 'name' does not exist on type 'User'.
5 this.name = name
~~~~
[8:31:56 PM] Found 1 error. Watching for file changes.
This indicates that although the class is valid in JavaScript, we need to define the name property in typescript.