Methods of most important arrays in JavaScript (filter, map, ...)
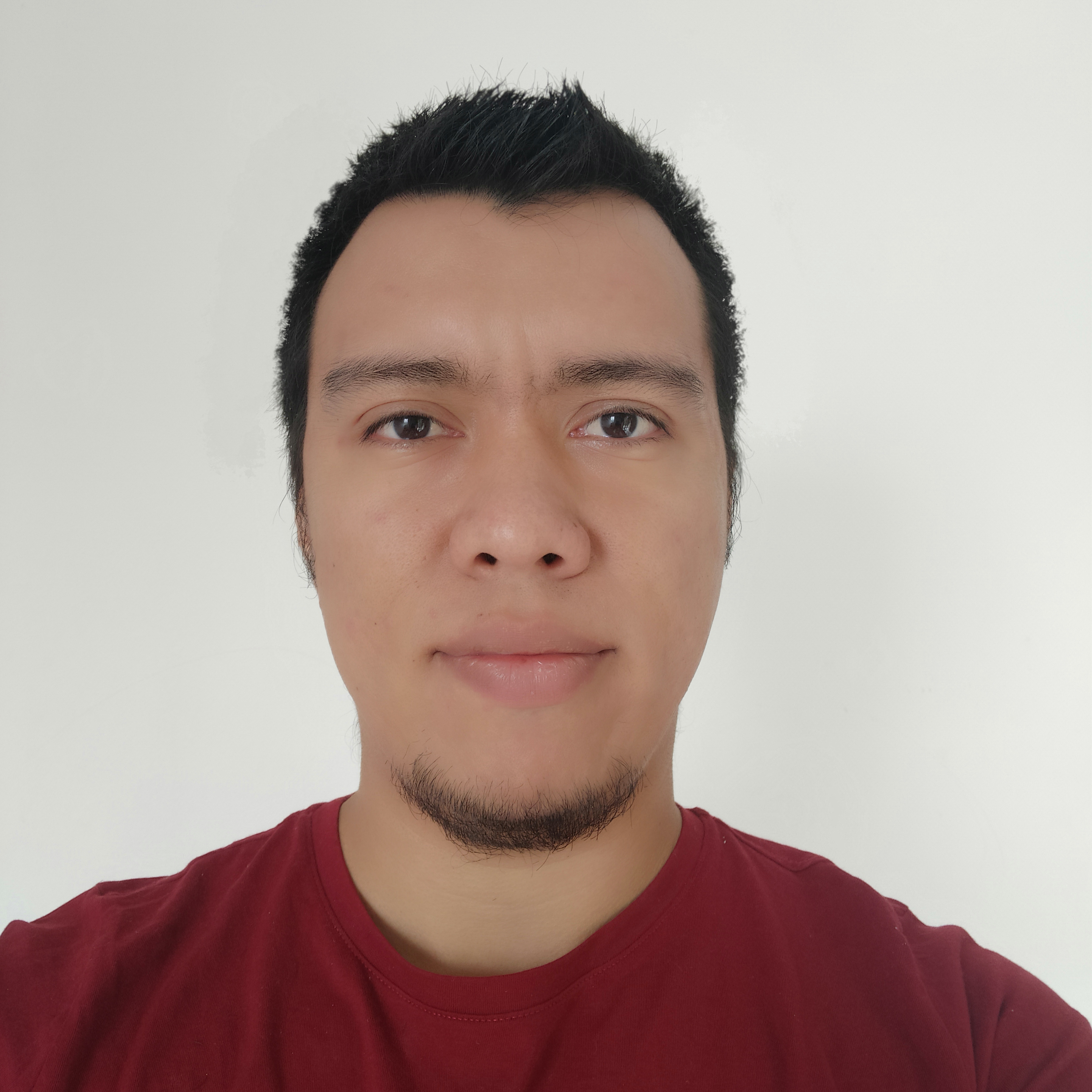
One of the most important aspects when working with arrays in javascript is the broad and coherent range of methods that exist to manipulate the data. In this post, we will see the most important methods of arrays in javascript.
In the following image taken from reddit, the most important methods in javascript and their application are summarized. It's quite intuitive!
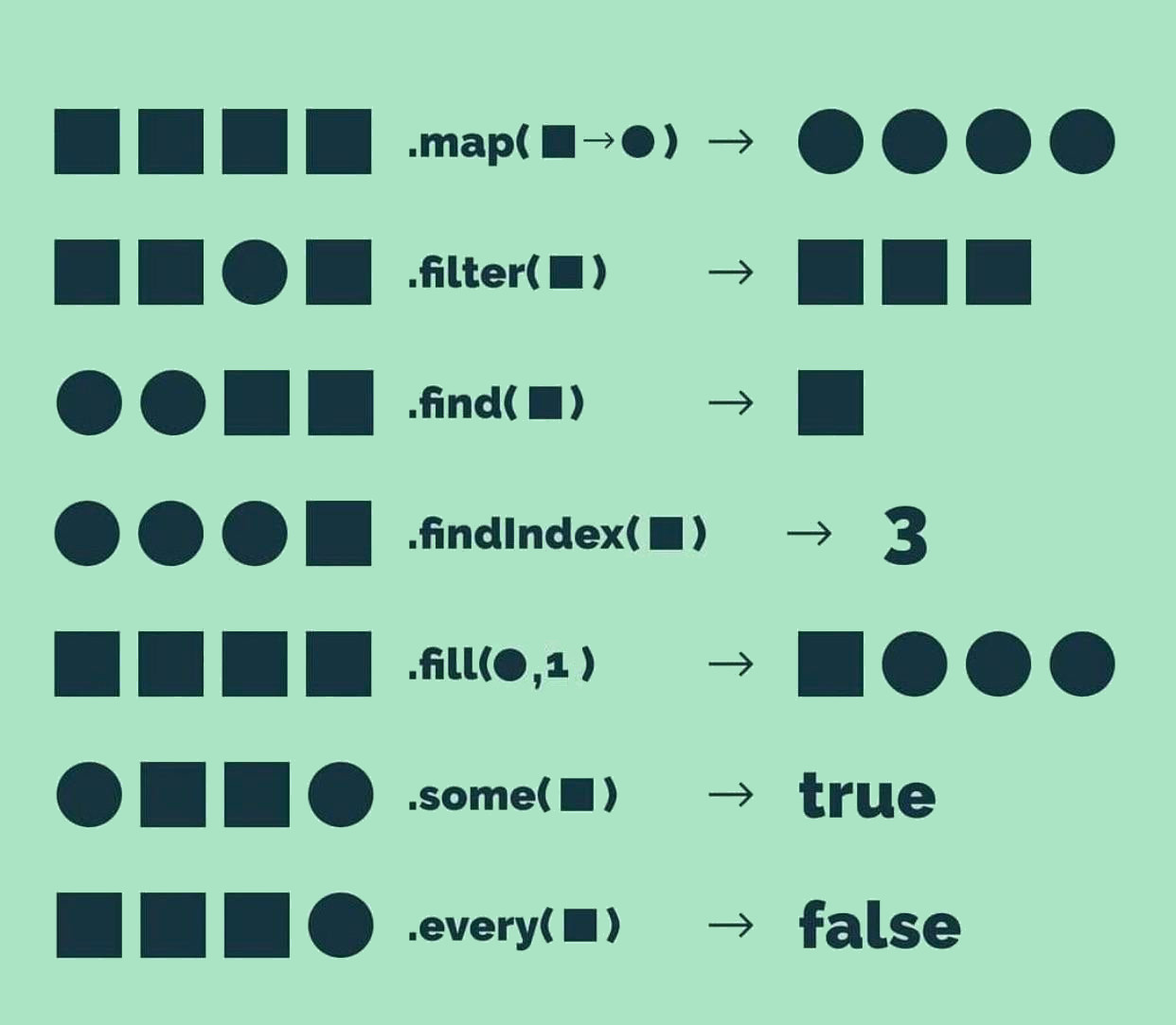
map
This method creates a new array from each element of the original array.
const numbers = [1,2,3]
const squares = numbers.map(number => {
return number * number
})
console.log(squares)
The result would be as follows:
[1,4,9]
In objects, it is commonly used to do a pluck of some property.
const devs = [
{name: 'Steave', role: 'frontend'},
{name: 'Andrew', role: 'backend'}
]
const names = devs.map(dev => {
return dev.name
})
console.log(names)
The result would be as follows:
['Steave', 'Andrew']
Another common use is to modify one or more properties of the original array. This, in fact, is not considered a good practice because it contradicts one of the principles of functional programming and because modifying the original array does not make sense to return a new one.
const devs = [
{name: 'Steave', role: 'frontend'},
{name: 'Andrew', role: 'backend'}
]
const allDevs = devs.map(dev => {
dev.role = 'backend'
return dev
})
console.log(devs)
The counterpart of the previous example is to return a new array with some modified properties if the original is not altered.
const devs = [
{name: 'Steave', role: 'frontend'},
{name: 'Andrew', role: 'backend'}
]
const allDevs = devs.map(dev => {
return {
...dev,
role: 'backend'
}
})
console.log(devs)
filter
This method creates a new array with all elements that pass the test in the function condition.
const devs = [
{name: 'Steave', role: 'frontend', age: 24},
{name: 'Andrew', role: 'backend', age: 28},
{name: 'James', role: 'backend', age: 17}
]
const filtered = devs.filter(dev => {
return dev.age > 20
})
console.log(filtered)
The result would be as follows:
[
{name: 'Steave', role: 'frontend', age: 24},
{name: 'Andrew', role: 'backend', age: 28},
]
In these terms, we can say that the result is a subset of the main array.
find
This method returns the element of the array that meets the condition given by the function.
const devs = [
{name: 'Steave', role: 'frontend', age: 24},
{name: 'Andrew', role: 'backend', age: 28},
{name: 'James', role: 'backend', age: 17}
]
const filtered = devs.find(dev => {
return dev.age === 17
})
console.log(filtered)
The result would be as follows:
{name: 'James', role: 'backend', age: 17}
findIndex
This method returns the index of the first element that meets the condition provided in the function. If it does not find any element, it returns -1.
const devs = [
{name: 'Steave', role: 'frontend', age: 24},
{name: 'Andrew', role: 'backend', age: 28},
{name: 'James', role: 'backend', age: 17}
]
const index = devs.findIndex(dev => {
return dev.age < 20
})
console.log(index)
The result would be as follows:
2
fill
This method changes all indicated elements in the array for a static value and returns the modified array.
const devs = ['Steave', 'Andrew', 'James', 'Bashir']
const cleaned = devs.fill(null, 1, 3)
console.log(cleaned)
The result would be as follows. The script fills the results from position 1 to 3 without including the latter.
['Steave', null, null, 'Bashir']
some
This method returns true
if at least one of the elements in the array passes the validation of the function.
const devs = [
{name: 'Steave', role: 'frontend', age: 24},
{name: 'Andrew', role: 'backend', age: 28},
{name: 'James', role: 'backend', age: 17}
]
const some = devs.some(dev => {
return dev.age < 20
})
console.log(some)
The result would be as follows:
true
every
This method returns true
if all elements in the array pass the validation of the function.
const devs = [
{name: 'Steave', role: 'frontend', age: 24},
{name: 'Andrew', role: 'backend', age: 28},
{name: 'James', role: 'backend', age: 17}
]
const every = devs.every(dev => {
return dev.age < 20
})
console.log(every)
The result would be as follows:
false