Laravel MVC pattern
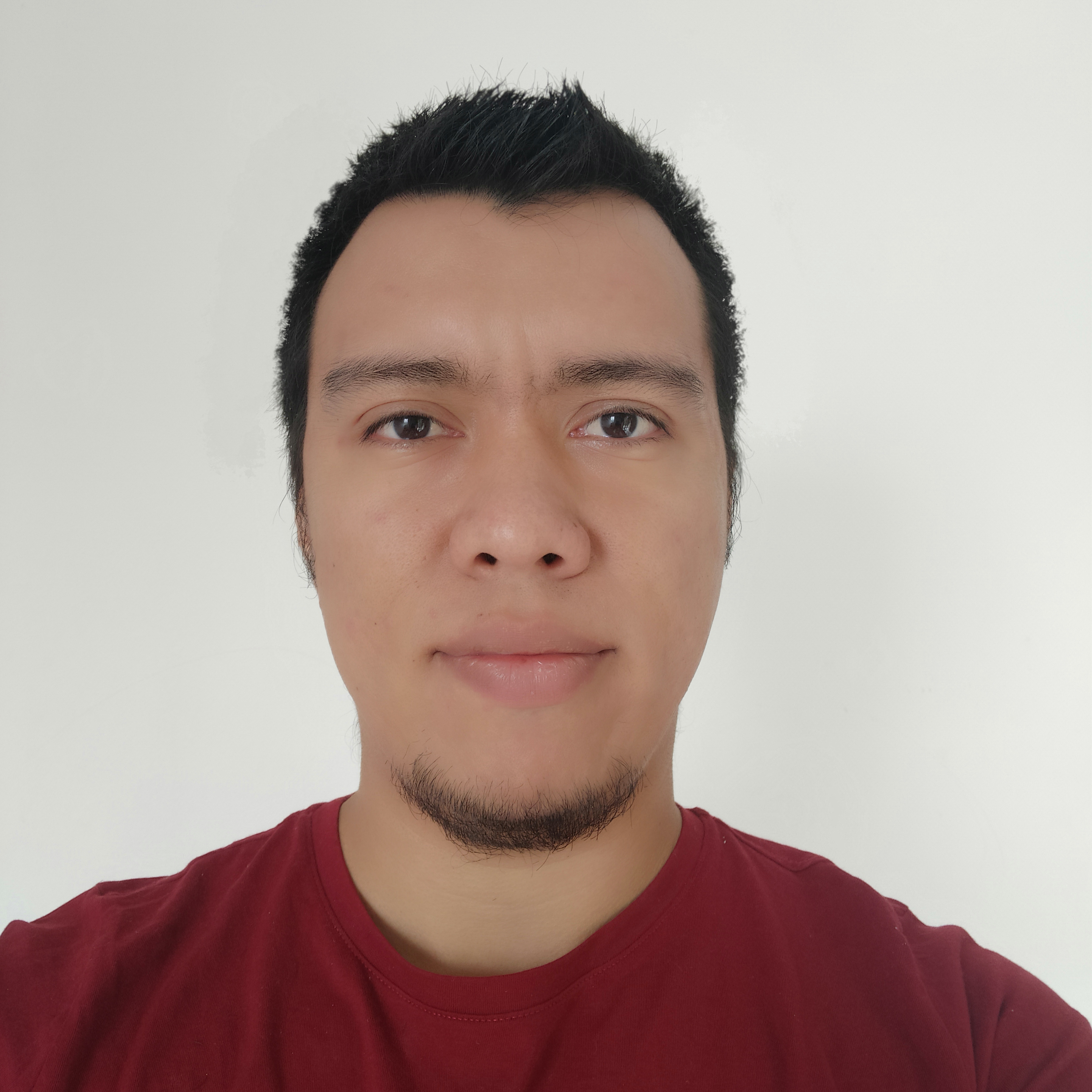
MVC (model, view, controller) is a software architectural pattern that separates an application into three layers as its acronym indicates. Laravel, as well as most frameworks in PHP, implement this design pattern where each layer handles a aspect of the application. However, before seeing how Laravel is designed to implement this software pattern, we will try to clarify this concept a little more by defining each of its parts..
Model: Refers to the data structure of the application. Data can be transferred from the database, a class, a service, or others, directly to the view or be transformed in the controller to be updated again at the source.
View: It is the representation of the information in a user interface. Usually in non-static interfaces, data that come directly from the model or these are transformed in an intermediate process in the controller are represented. In static views, it is usually unnecessary for views to be rendered with data sent from the controller.
Controller: It is the place where the application logic, procedures, algorithms, and routines that make the software work are implemented. Acts as an interface between model and view components by applying necessary transformations and logic.
MVC in Laravel
MVC in Laravel is implemented as follows. In a web application, the controllers will be located in the folder app/Http/Controllers
, models directly in app
and the views in resources/views
. To create a new controller, just run the command
php artisan make:controller HelloWorld
This command will create the file app/Http/Controllers/HelloWorld.php which will have the following content.
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class HelloWorld extends Controller
{
//
}
Of course, if you know this structure beforehand, you can create the controller yourself. For me, it is much more dynamic to create it from artisan and I recommend that you always use this command provided by Laravel. As you can see, this command created a controller that inherits directly from the class App\Http\Controllers
of Laravel. To make our controller functional, we will add the following method.
public function sayHello()
{
return view('hello');
}
Methods in laravel controllers usually return arrays, objects or views. In this case, we have used the helper view
to return the hello view which we will create below.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<title>App title</title>
<body>
<h1>HELLO WORLD!</h1>
</body>
</html>
This file should be saved with the name hello.blade.php in the resources/views folder. Finally, we will add the following line in the file routes/web.php to be able to access the sayHello
method which will return the hello view.
Route::get('/helloworld', 'HelloWorld@sayHello');
When accessing the route /helloworld along with the root domain of your application in the browser, the message HELLO WORLD! should be displayed. You can try this before continuing with what comes next.
To add the model component, we will run the following command in the terminal
php artisan make:model Hello
This will create the file app/Hello.php with the following content
<?php
namespace App;
use Illuminate\Database\Eloquent\Model;
class Hello extends Model
{
//
}
After this, we will add the following method to our model to return the greeting.
public static function helloWorldMessage()
{
return "HELLO WORLD!";
}
Next, we will make the following change in the controller to send the message from the model to the view.
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Hello; // here we import the model
class HelloWorld extends Controller
{
public function sayHello()
{
return view('hello', ["message" => Hello::helloWorldMessage()]);
}
}
Finally, we will make the following change in the view to print the message sent by the controller.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<title>App title</title>
<body>
<h1> {{ '\{\{ $message \}\}' }} </h1>
</body>
</html>
And with this, when accessing the route /helloworld again, we will see the HELLO WORLD! message brought directly from our model. Until next time!.