Operators at the bit level in C
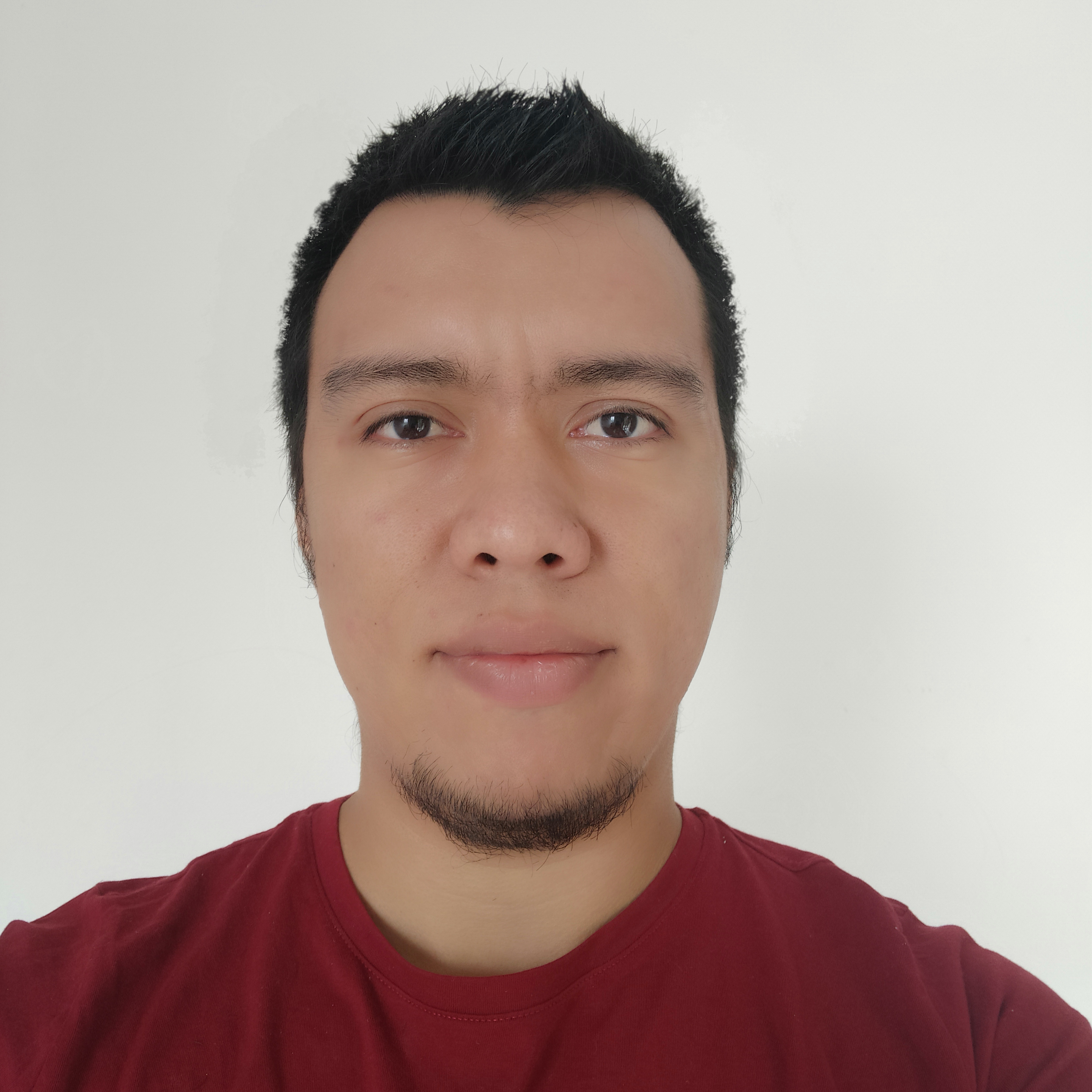
Bitwise operators allow you to perform shifts and certain bit operations. These operations can only be performed on integers and these are treated more as sets of bits than as decimal numbers.
At the beginning, performing bitwise operations may seem a little confusing since shifting one bit from a number like 65 can result in doubling or halving that number. However, simply observing the movement of the bits and understanding their binary and decimal representation is enough.
For the following examples, let's assume that the type short
has a size of 16 bits. With this assumption, we are going to represent some numbers and perform operations on them. I recommend you read our article Data types in C first, as it explains how data types are created and why they have a specified number of bits.
Operator <<
The left shift operator ( <<
) moves each bit to the left and places a zero as the least significant bit (farthest to the right), and the most significant bit (farthest to the left) is discarded. Observe the following example where this operator is used to shift one bit to a number, resulting in its double (decimal).
Operation
<< 0000000001000001 (65)
0000000010000010 (130)
Code
short a = 65;
printf("Dec: %d\n\n", a);
a = a << 1;
printf("Dec: %d\n", a);
Output
Dec: 65
Dec: 130
Operator >>
The right shift operator ( >>
) moves each bit to the right discarding the less significant bits. Of course, the most significant bits are completed with zeros. Observe the following example where this operator is used to shift one bit to a number, resulting in its half (decimal).
Operation
<< 0000000000001010 (10)
0000000000000101 (5)
Code
short a = 10;
printf("Dec: %d\n\n", a);
a = a >> 1;
printf("Dec: %d\n", a);
Output
Dec: 10
Dec: 5
Operator & (AND)
The AND operator ( &
) compares two bits. If both are 1, then the result will be 1, otherwise the result will be zero. Observe the following example where we perform this bit-by-bit comparison of two numbers.
Operation
& 0000000010101010 (170)
0000000011000000 (192)
Result:
0000000010000000 (128)
Code
short a = 170;
short b = 192;
short c = a & b;
printf("Operation: %d & %d\n", a, b);
printf("Result: %d\n", c);
Output
Operation: 170 & 192
Result: 128
Operator | (OR)
The OR operator ( |
) compares two bits. If at least one of them is 1, then the result will be 1, otherwise the result will be zero. Observe the following example where we perform this bit-by-bit comparison of two numbers.
Operation
& 0000000010101010 (170)
0000000011000000 (192)
Result:
0000000011101010 (234)
Code
short a = 170;
short b = 192;
short c = a & b;
printf("Operation: %d | %d\n", a, b);
printf("Result: %d\n", c);
Output
Operation: 170 & 192
Result: 134
Operator ^ (XOR)
The XOR operator ( ^
) compares two bits. If the two bits are complementary, then the result will be 1, otherwise the result will be zero. Observe the following example where we perform this bit-by-bit comparison of two numbers.
Operation
& 0000000010101010 (170)
0000000011000000 (192)
Result:
0000000001101010 (106)
Code
short a = 170;
short b = 192;
short c = a ^ b;
printf("Operation: %d ^ %d\n", a, b);
printf("Result: %d\n", c);
Output
Operation: 170 & 192
Result: 106