PHPUnit Installation
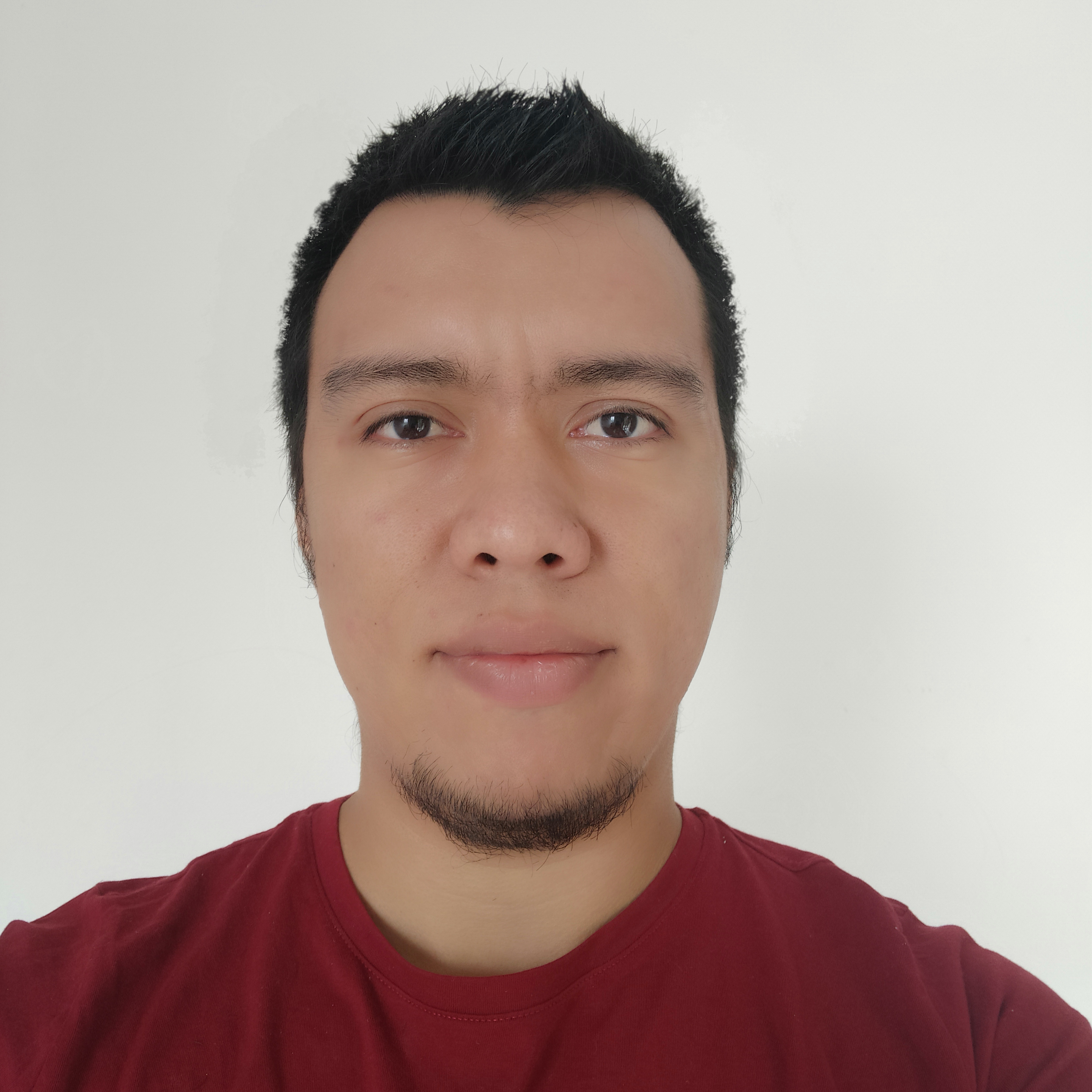
PHPUnit has proven to be for years the unit testing environment par excellence for PHP. For those who still do not know what unit tests are, a unit test is a software testing method to check that a certain software unit (function or class) works as expected. That is, it should throw the values it should throw, of the type they should be or throw the exceptions that it should throw for out-of-range values, etc. Today, we will see how to implement PHPUnit in our PHP projects regardless of whether we use a framework or not, or what type of framework we use.
Testing scenario
Let's make it interesting and assume that we have created the following class in PHP that performs the summation over a function defined from i to n. As you can see, this class consists only of a recursive function, with its base case and its call to itself.
class Summation
{
public function compute($start, $end, $function)
{
if ($start == $end)
return call_user_func($function, $end);
return call_user_func($function, $start) + $this->compute($start + 1, $end, $function);
}
}
So let's perform unit tests and ensure the correct functioning of this class. First of all, let's save this class in a file called Summation.php. Usually for unit tests a folder called test or tests is created within the project root. So let's create a folder called test and integrate PHPUnit.
Installation without composer
This way of installing PHPunit involves downloading the package in phar format. To do this, we are going to open a shell in the folder where our project is located and we are going to execute the following command:
wget -O phpunit https://phar.phpunit.de/phpunit-8.phar
chmod u+x phpunit
If you use Windows you can download the file by pasting the URL directly into the browser. As a result of this command we will have the phpunit file in our directory.
Installation with composer
If you have composer installed globally you can install PHPUnit by running the following command in console located in the project directory.
composer require --dev phpunit/phpunit ^8.3
Test design
To perform our unit test we are going to create the SummationTest.php file in the test directory and we are going to add the following content:
namespace Test;
Use Summation;
use PHPUnit\Framework\TestCase;
class SummationTest extends TestCase
{
public function testItSumsAConstant()
{
$summation = new Summation();
$res = $summation->compute(1, 5, function() { return 10; });
$this->assertEquals(5 * 10, $res);
}
public function testItSumsWithTheIdentityFunction()
{
$summation = new Summation();
$res = $summation->compute(1, 5, function($x) { return $x; });
$this->assertEquals(1 + 2 + 3 + 4 + 5, $res);
}
}
The previous class has two tests each with an assertion. The first test checks that our compute class correctly computes the sum from 1 to n of a constant, the second test checks that the sum of the first n natural numbers is computed correctly, specifically, the first five. To run unit tests, simply run the following command in the console:
./phpunit --bootstrap Summation.php test
If you installed PHPUnit with composer you just have to change the path of the phpunit binary like this:
./vendor/bin/phpunit --bootstrap Summation.php test
If everything went well, this command will display an output similar to the following:
PHPUnit 8.3.4 by Sebastian Bergmann and contributors.
.. 2 / 2 (100%)
Time: 61 ms, Memory: 10.00 MB
OK (2 tests, 2 assertions)
Later we will see how to perform all types of tests on a PHP project. See you soon!