Program Structure in GO
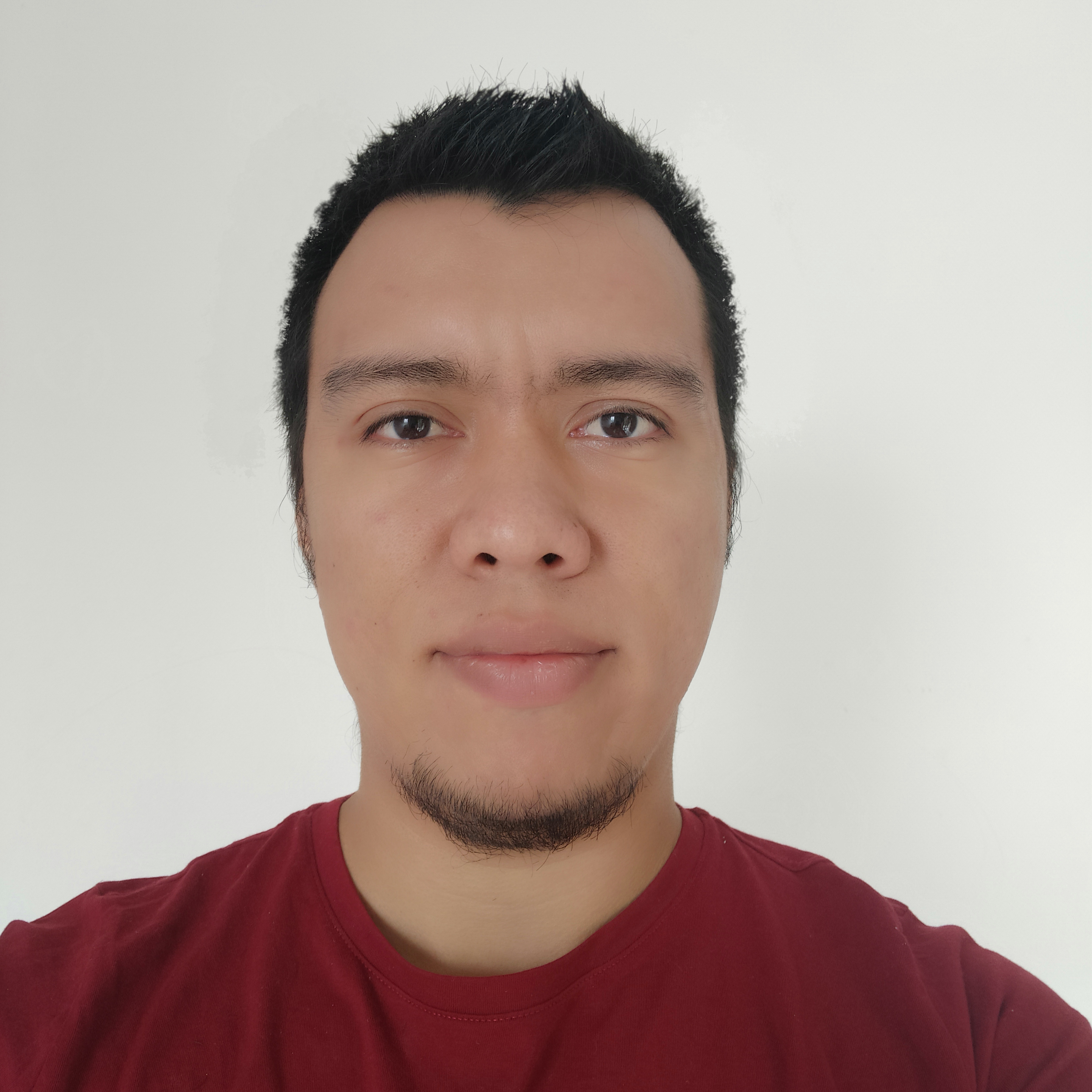
In a previous post we saw the installation of Go in Linux, today let's see what elements we need to make any program in Go.
Initial structure
All Go programs start with the main
function in the main
package. Additionally, it is not necessary to end statements with ;
(semicolon).
package main
import "fmt"
func main() {
fmt.Printf("hello, world\n")
}
In the previous program, we can see that the first section of the program composed of the first line contains the declaration of the current package
, which in our case is main
. The second section of the program composed of the third line contains the declaration of the import paths that uses the reserved word import
. Import paths basically load libraries (classes) from the core or from third parties. The third part of the program composed of the last three lines defines the main
function which is the starting point of the program. To add more than one import path, you can add as many lines as needed.
import "fmt"
import "math/rand"
Or use the recommended syntax (factored).
import (
"fmt"
"math/rand"
)
Variables
The initial structure seen above is the initial scaffold for creating programs in Go. However, a program without variables is of little use. Variables in Go can be defined at both the package and function level.
package main
import "fmt"
var c, python, java bool
func main() {
var i int
fmt.Println(i, c, python, java)
}
Variables can be declared and initialized in the same statement as shown below.
var i, j int = 1, 2
When using the initializer, the variable type can be omitted, leaving it as follows:
var i, j = 1, 2
It is also possible to declare and initialize variables of different types.
var c, python, java = true, false, "no!"
If the variable declaration depends on an expression or is composed of many statements, the factored or block form can be used as follows:
var (
isSomething bool = false
MaxInt uint64 = 1<<64 - 1
z complex128 = cmplx.Sqrt(-5 + 12i)
)
Finally, within a function it is possible to avoid the use of the reserved word var
in the definition of variables by combining it with the short assignment declaration :=
.
k := 3
c, python, java := true, false, "no!"
See you next time!.