Resource controllers in Laravel
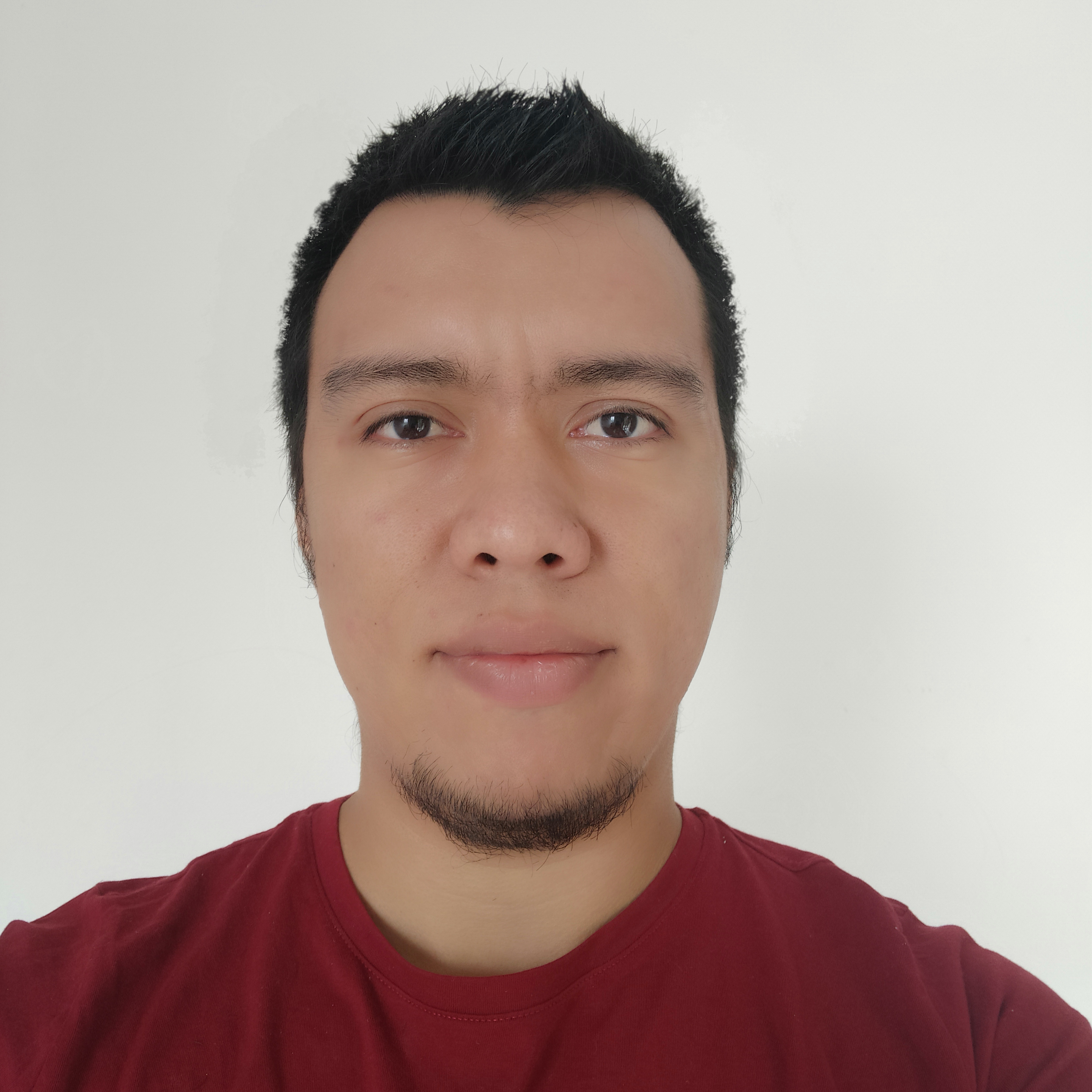
So far, everything we have seen from day one on this blog is what I consider the basic level in Laravel. In a previous post, we covered the topic of Laravel controllers, Today, we will explore a slightly deeper topic that I would consider to be at an intermediate level. Not because of the complexity of the topic itself, but because many developers tend to stick with the basic knowledge we have covered previously. Without further ado, let's dive into the resource controllers.
What is a resource controller?
A resource controller is a controller that includes the typical CRUD operations (create, read, update, delete). These controllers often come into play when designing an interface for managing or administering entities within your application, such as the Users entity. When you create a controller that manages users, whether it's called UsersController, AdminUsers, or any other name, you typically have methods that define actions on this entity, such as view (show), update, create, and delete. A resource controller in Laravel helps you with the scaffolding of these controllers to create and manage them more efficiently.
Creating a resource controller
To create a resourcecontroller, you need to execute the following command with Artisan:
php artisan make:controller UsersController --resource
This command will create a scaffold for the controller similar to the following:
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class UsersController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
//
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
//
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
//
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
//
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit($id)
{
//
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
//
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
//
}
}
Next, the next thing we need to do to make our resource controller fully functional is to define the routes in the routes/web.php file. However, instead of defining each route for each method individually, we can do it in the following way:
Route::resource('users', 'UsersController');
We will see how the routes are interpreted from the browser next.
Resource controller actions
The base table to understand how routes are interpreted is as follows:
Verbo | URI | Acción | Ruta |
---|---|---|---|
GET | /users | index | users.index |
GET | /users/create | create | users.create |
POST | /users | store | users.store |
GET | /users/{photo} | show | users.show |
GET | /users/{photo}/edit | edit | users.edit |
PUT/PATCH | /users/{photo} | update | users.update |
DELETE | /users/{photo} | destroy | users.destroy |
The interpretation is simple and predictable. For example, if you want to display the information of a specific user (READ), you should use the route name users.show (GET), which implies implementing the necessary logic in the show method. If you want to create a new user, you can use the route users.create (GET) to display the user creation form, and the route users.store (POST) to process the creation of the record.
If you haven't noticed yet, you may wonder how to process a record using PUT/PATCH/DELETE by sending the request from an HTML form. Laravel solves this by using Blade to create an additional data in the form that indicates the method to use.
<form>
@method('PUT')
</form>
In addition to this, you can partially define the routes you are going to use if you don't need all of them. For example, if you only need the index
and show
actions, you should define the route for the resource controller as follows:
Route::resource('users', 'UsersController')->only([
'index', 'show'
]);
If, on the contrary, you need to specify which routes you won't be using, you can simply change the only
method to except
.
Route::resource('users', 'UsersController')->except([
'create', 'store', 'update', 'destroy'
]);
I hope you have learned a bit more with this post and that you can implement this type of controllers in your Laravel applications as soon as possible. See you soon!.