Build a REST API with Laravel
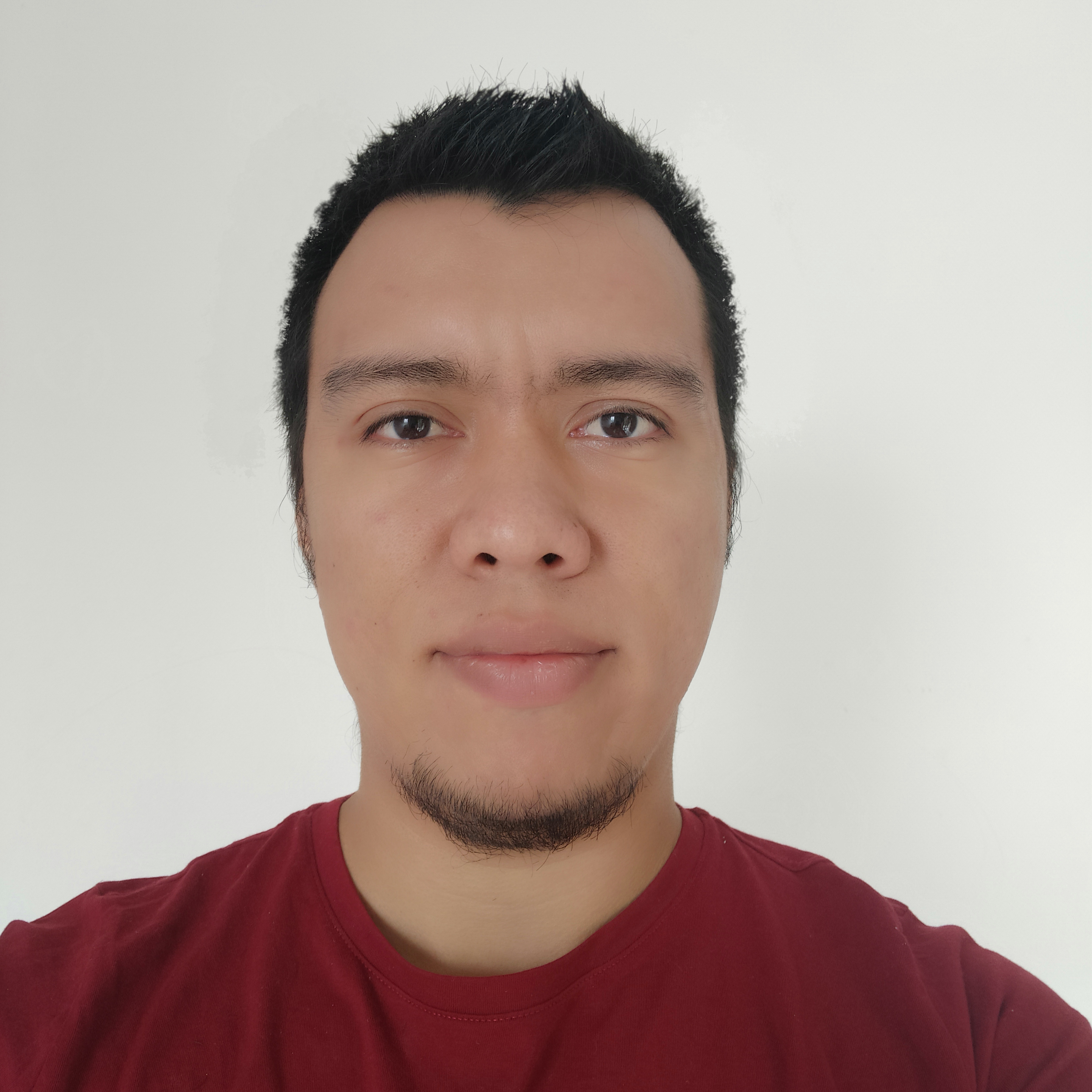
Creating a REST API is a relatively simple and rewarding task in Laravel. That's why today you will learn how to create a RESTful web service using an API built with Laravel. If you still have some conceptual gaps about RESTful systems, we recommend visiting our previous post on REST APIs, After that, you will be an expert in no time.
Create the Project
The first thing you need to do is to install a new Laravel project using the following command:
laravel new api-rest
If you don't have the "laravel" command enabled on your computer yet, we recommend visiting our post Laravel Installation To cover everything related to the installation and setup of an environment for Laravel.
Create the API Controller
For the API controller, we will use a resource controller since it already includes the necessary methods and HTTP verbs we need. You can find more information about this type of controllers in our post. Resource Controllers in Laravel.
php artisan make:controller ApiController --resource
The next step is to implement the CRUD functionalities in the controller. For this specific example, we will omit the database layer and make modifications to a plain file to simplify the exercise. The goal of this controller is to demonstrate the basic functionality of a REST API, so many validations, refactoring, and the PSR standard are omitted. Our controller would look like this.
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Storage;
class ApiController extends Controller
{
public function __construct()
{
if (!Storage::disk('local')->exists('file.txt'))
Storage::disk('local')->put('file.txt', '');
}
private function toArray($data)
{
$parsed = [];
foreach ($data as $line) {
$row = explode(',', $line);
if (count($row) === 3)
$parsed[$row[0]] = [
'code' => $row[0],
'language' => $row[1],
'developer' => $row[2],
];
}
return $parsed;
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
$line = $request->input('code') . ',' .
$request->input('language') . ',' .
$request->input('developer');
$response = Storage::disk('local')->prepend('file.txt', $line);
return response()->json([
'status' => ($response) ? 'created' : 'failed'
]);
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
$data = explode(PHP_EOL, Storage::disk('local')->get('file.txt'));
$languages = $this->toArray($data);
$response = [
'status' => 'not found'
];
if (in_array(1, array_column($languages, 'code'))) {
foreach ($languages as $key => $language) {
if ($id == $key) {
$response = $language;
break;
}
}
}
return response()->json($response);
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
$data = explode(PHP_EOL, Storage::disk('local')->get('file.txt'));
$languages = $this->toArray($data);
foreach ($languages as $key => $language) {
if ($id == $key) {
$line = [
'code' => $language['code'],
'language' => $request->input('language'),
'developer' => $request->input('developer')
];
$languages[$key] = $line;
break;
}
}
foreach ($languages as $key => $language) {
$languages[$key] = implode(',', array_values($language));
}
$response = Storage::disk('local')->update('file.txt', implode(PHP_EOL, $languages));
return response()->json([
'status' => ($response) ? 'updated' : 'failed'
]);
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
$data = explode(PHP_EOL, Storage::disk('local')->get('file.txt'));
$languages = $this->toArray($data);
foreach ($languages as $key => $language) {
if ($id == $key) {
unset($languages[$key]);
break;
}
}
foreach ($languages as $key => $language) {
$languages[$key] = implode(',', array_values($language));
}
$response = Storage::disk('local')->update('file.txt', implode(PHP_EOL, $languages));
return response()->json([
'status' => ($response) ? 'deleted' : 'failed'
]);
}
}
Create the route
Finally, we just need to create the route to access the API in the routes file.
Route::resource('file', 'ApiController');
With this, we can now proceed to test our API. For flexibility, we have conducted the tests by running the server using artisan.
php artisan serve
Test the API
To test the API, we can use Postman or any other software for API development.
Create a record
To create a record, we will make the following request to the API.
POST http://127.0.0.1:8000/api/file
{
"code": 1,
"language": "PHP",
"developer": "Dennis Ritchie"
}
The result should be as follows:
{
"status": "created"
}
Update a record
To update the previously created record, we will make the following request to the API.
PUT/PATCH http://127.0.0.1:8000/api/file/1
{
"language": "PHP",
"developer": "Rasmus Lerdorf"
}
The result should be as follows:
{
"status": "updated"
}
Retrieve a record
To retrieve a record, we will make the following request to the API.
GET http://127.0.0.1:8000/api/file/1
The result should be as follows:
{
"code": 1,
"language": "PHP",
"developer": "Rasmus Lerdorf"
}
Delete a record
To delete a record, we will make the following request to the API.
DELETE http://127.0.0.1:8000/api/file/1
The result should be as follows:
{
"status": "deleted"
}