Iteration Statements in JavaScript (for)
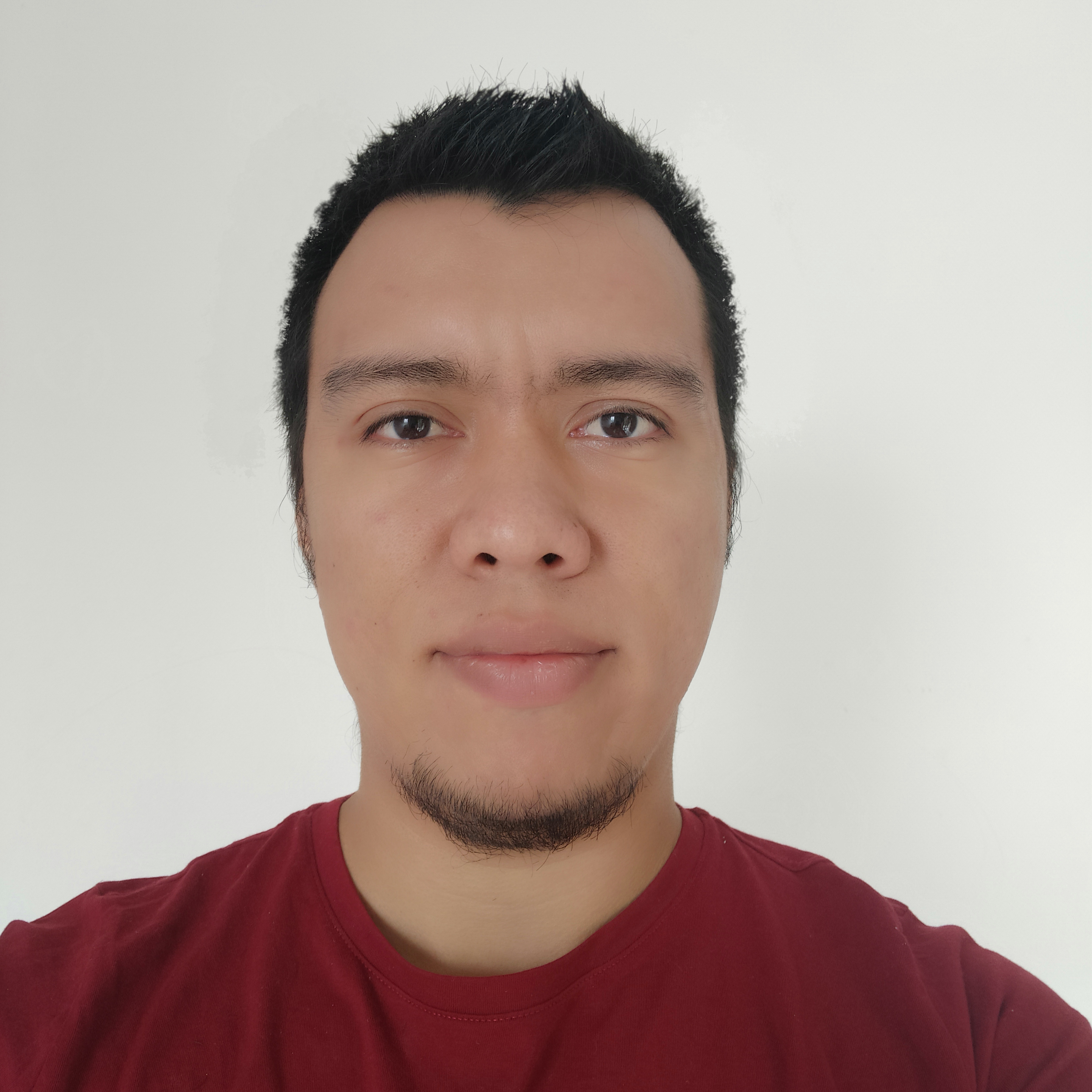
By
Darío Rivera
Posted On
in
Javascript
In a previous post we saw the switch decision statement in javascript. Today we will begin to see the iteration statements and the first one we will see is everything related to the for statement. Iterative statements allow you to execute a block of code as many times as necessary. Let's see how these statements work.
for
The traditional way to use the for statement on an array is as follows:
const items = ['foo', 'bar', 'baz']
for (let i = 0; i < items.length; i++) {
console.log(`Item: ${items[i]}`)
}
The output of this script is below:
Item: foo
Item: bar
Item: baz
for-of
A more modern way to iterate an array is as follows:
const items = ['foo', 'bar', 'baz']
for (const item of items) {
console.log(`Item: ${items[i]}`)
}
forEach
This way is much more straightforward and is the preferred way to iterate arrays in JavaScript.
const items = ['foo', 'bar', 'baz']
items.forEach(item => {
console.log(`Item: ${item}`)
})
items.forEach((item, index) => {
console.log(`Item ${index}: ${item}`)
})
for-in
This loop allows you to iterate over objects instead of arrays. The iteration will be performed on each property of the object.
const items = {a: 'foo', b: 'bar', c: 'baz'}
for (item in items) {
console.log(`Item: ${items[item]}`)
}