Slots in Vue.js
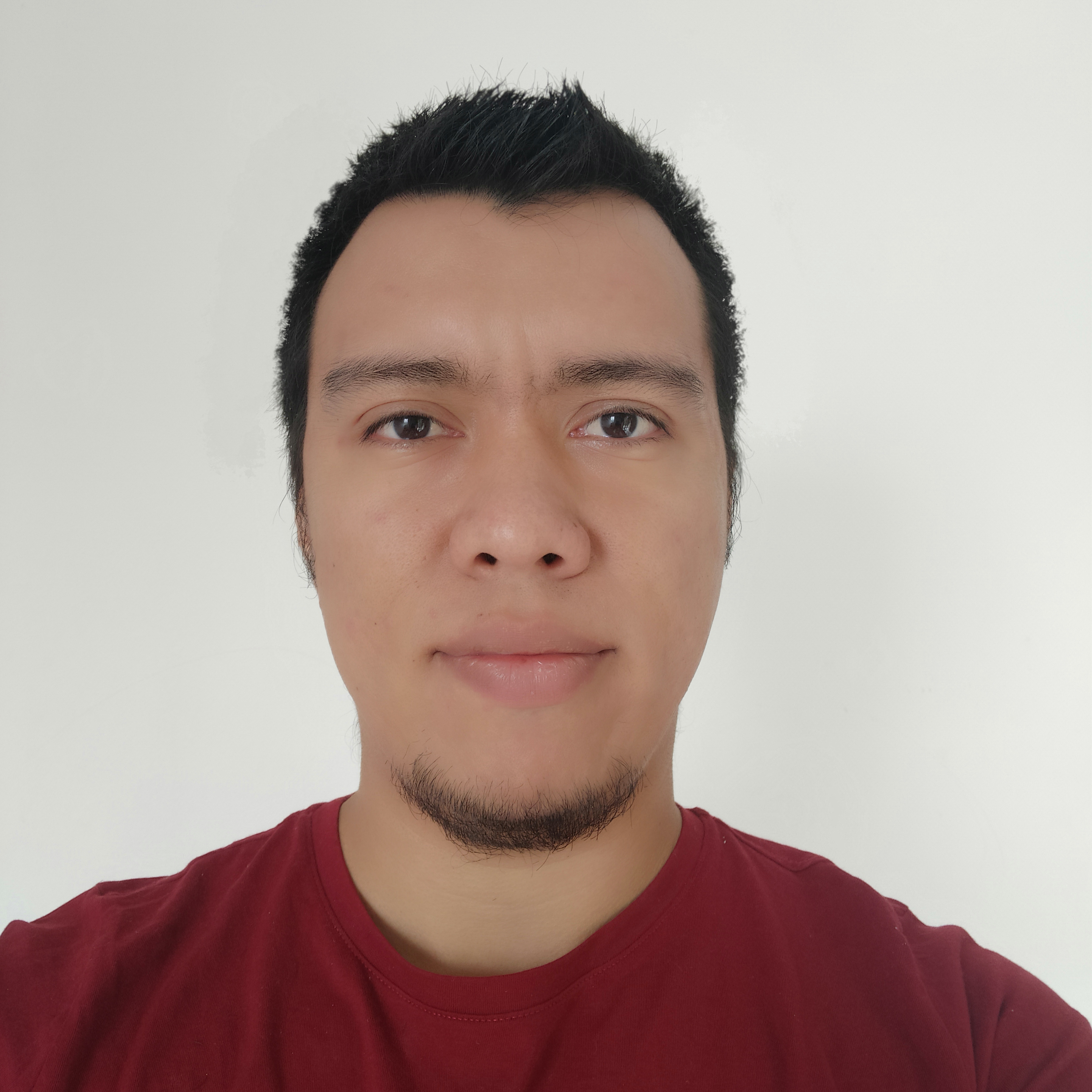
The concept of Slot is difficult to define but easy to understand by seeing a few examples. A slot is like a component fragment to which content can be injected. This allows us to use Vue components in the same way as an HTML block element allowing other elements to be nested inside it.
<div id="app" class="m-2">
<x-button type="danger">
<strong>Click</strong>
for more info!
</x-button>
</div>
Notice how in the above HTML we use the strong
element inside our x-button
component. Let's take a closer look at this below.
Slots
The content of a component within the tags that define it is by default a slot. In the previous example, the slot would be the following:
<strong>Click</strong>
for more info!
To inject this content into the component, it is necessary to use the slot
tag in the template definition.
Vue.component('x-button', {
props: ['type'],
computed: {
className: function() {
return `btn-${this.type}`;
},
},
template: `
<button
type="button"
:class="['btn', className]"
@click="$emit('click', $event)"
>
<slot></slot>
</button>
`
})
var vue = new Vue({
el: "#app"
});
Default content of a Slot
We can change the above code a bit and use the default content of the slot to display default text on the button. This is achieved by adding said content inside the slot
tag.
<button
type="button"
:class="['btn', className]"
@click="$emit('click', $event)"
>
<slot>Action</slot>
</button>
If no content is defined inside our component, the default content of the slot will be rendered. You can see the example on codepen below.
It is also possible to have more than one slot in the same component. Check out our article Named Slots in Vue.js. See you next time!.