Size of Data Types in C
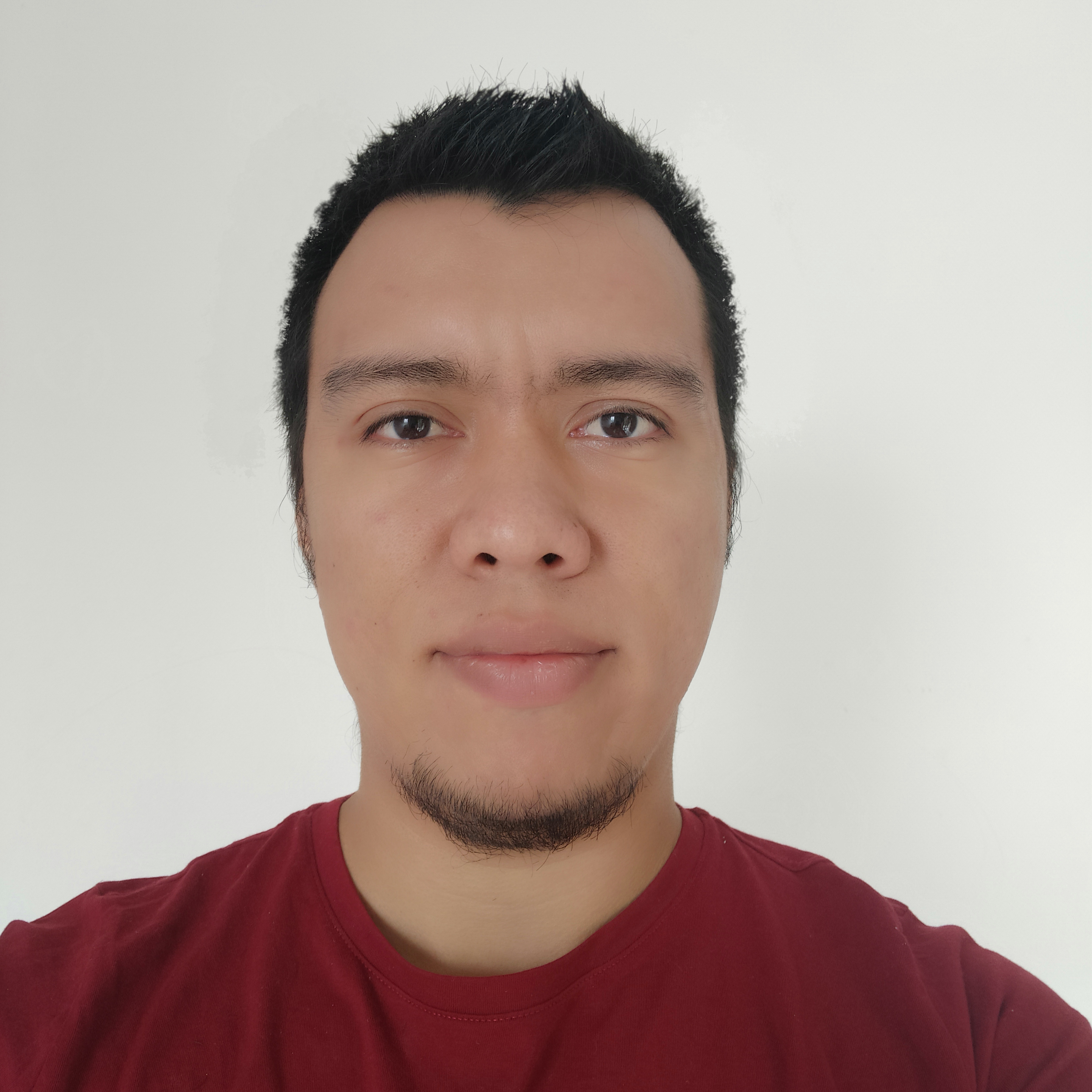
By
Darío Rivera
Posted On
in
Lenguaje C
In our previous article we saw all the Data Types in C and in particular the char data type. Each data type has a specific bit storage that can be calculated with the sizeof function. Some values may vary depending on the machine where C is being used.
Below is a program that shows in detail all the sizes of the primitive types.
#include <stdio.h>
#include <limits.h>
#include <float.h>
int main()
{
printf("+--------+-----------+------------------------------------------------------+\n");
printf("| TYPE | SIZE | RANGE \n");
printf("+--------+-----------+------------------------------------------------------+\n");
printf("| char | %d byte | (signed) %d to %d \n", CHAR_BIT/8, CHAR_MIN, CHAR_MAX);
printf("| | | (unsigned) 0 to %d \n", UCHAR_MAX);
printf("+--------+-----------+------------------------------------------------------+\n");
printf("| short | %lu bytes | (signed) %d to %d \n", sizeof(short), SHRT_MIN, SHRT_MAX);
printf("| | | (unsigned) 0 to %d \n", USHRT_MAX);
printf("+--------+-----------+------------------------------------------------------+\n");
printf("| int | %lu bytes | (signed) %d to %d \n", sizeof(int), INT_MIN, INT_MAX);
printf("| | | (unsigned) 0 to %ud \n", UINT_MAX);
printf("+--------+-----------+------------------------------------------------------+\n");
printf("| long | %lu bytes | (signed) %ld to %ld \n", sizeof(long), LONG_MIN, LONG_MAX);
printf("| | | (unsigned) 0 to %lu \n", ULONG_MAX);
printf("+--------+-----------+------------------------------------------------------+\n");
printf("| long | %lu bytes | (signed) %lld to %lld \n", sizeof(long long), LLONG_MIN, LLONG_MAX);
printf("| long | | (unsigned) 0 to %llu \n", ULLONG_MAX);
printf("+--------+-----------+------------------------------------------------------+\n");
printf("| float | %lu bytes | %g to %g \n", sizeof(float), FLT_MIN, FLT_MAX);
printf("| | | %g to %g \n", -FLT_MAX, -FLT_MIN);
printf("+--------+-----------+------------------------------------------------------+\n");
printf("| double | %lu bytes | %g to %g \n", sizeof(double), DBL_MIN, DBL_MAX);
printf("| | | %g to %g \n", -DBL_MAX, -DBL_MIN);
printf("+--------+-----------+------------------------------------------------------+\n");
printf("| long | %lu bytes | %g to %g \n", sizeof(long double), DBL_MIN, DBL_MAX);
printf("| double | | %g to %g \n", -DBL_MAX, -DBL_MIN);
printf("+--------+-----------+------------------------------------------------------+\n");
return 0;
}
When you compile and run this program, you will get output similar to the following:
+--------+-----------+------------------------------------------------------+
| TYPE | SIZE | RANGE
+--------+-----------+------------------------------------------------------+
| char | 1 byte | (signed) -128 to 127
| | | (unsigned) 0 to 255
+--------+-----------+------------------------------------------------------+
| short | 2 bytes | (signed) -32768 to 32767
| | | (unsigned) 0 to 65535
+--------+-----------+------------------------------------------------------+
| int | 4 bytes | (signed) -2147483648 to 2147483647
| | | (unsigned) 0 to 4294967295d
+--------+-----------+------------------------------------------------------+
| long | 8 bytes | (signed) -9223372036854775808 to 9223372036854775807
| | | (unsigned) 0 to 18446744073709551615
+--------+-----------+------------------------------------------------------+
| long | 8 bytes | (signed) -9223372036854775808 to 9223372036854775807
| long | | (unsigned) 0 to 18446744073709551615
+--------+-----------+------------------------------------------------------+
| float | 4 bytes | 1.17549e-38 to 3.40282e+38
| | | -3.40282e+38 to -1.17549e-38
+--------+-----------+------------------------------------------------------+
| double | 8 bytes | 2.22507e-308 to 1.79769e+308
| | | -1.79769e+308 to -2.22507e-308
+--------+-----------+------------------------------------------------------+
| long | 16 bytes | 2.22507e-308 to 1.79769e+308
| double | | -1.79769e+308 to -2.22507e-308
+--------+-----------+------------------------------------------------------+