Conversion of Data Types in PHP
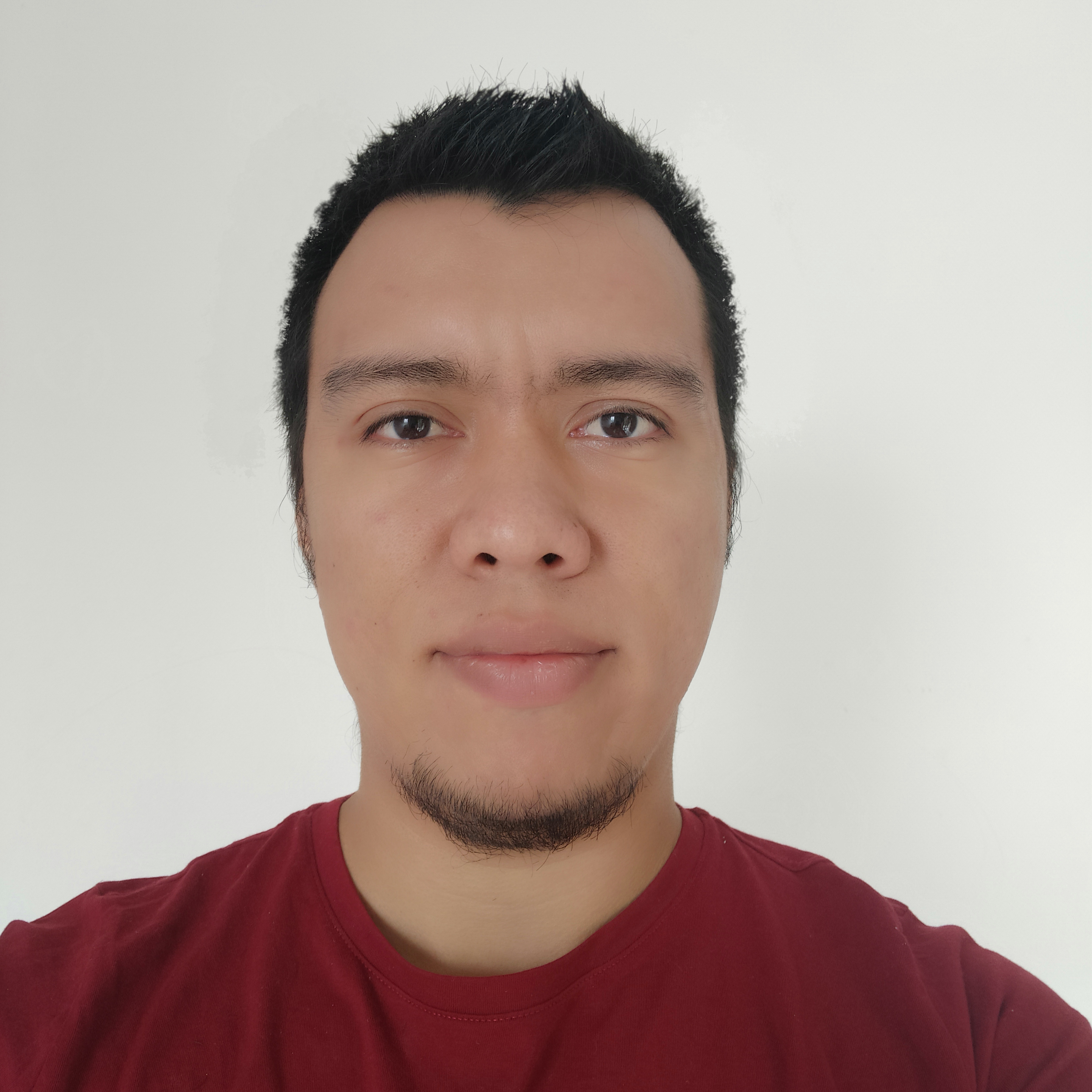
Remember that PHP is a weakly typed language, which means that it does not control the types of variables it declares. This way it is possible to use variables of any type in the same scenario. The type of a variable in PHP when it is not declared is determined by the context in which the variable will be used. Next we will see all the possible type conversions that we can make in PHP
Explicit Conversion
This type of conversion can be performed using type casting, and works in the same way as it does in the C language, by prefixing the desired type between parentheses just before the variable.
// this variable will be of type integer
$number = 1;
// this variable will be of type boolean
$bool = (boolean) $number;
echo gettype($bool); // shows "boolean"
Below you can see a table of all possible types of cast in PHP.
Expression | Final type |
---|---|
(int), (integer) | integer |
(bool), (boolean) | boolean |
(float), (double), (real) | float |
(string) | string |
(array) | array |
(object) | object |
Note that this type of conversion allows a variable to be evaluated with a specific type of data. To change the variable itself to a different type, there is the settype()
function.
// this variable is of type string
$height = "60.5";
// now we convert it to integer
settype($height, 'integer');
echo gettype($height); // shows "integer"
Automatic Conversion
Automatic type conversion is performed when a specific type of data is needed in an expression. Let's see the following example.
// this variable is of type double
$a = 1.5;
// this variable is of type integer
$b = 2;
// the variable $c will be of type double
$c = $a * $b;
echo gettype($c); // shows "double"
PHP internally evaluates the variable $a
as double to be able to multiply it with the variable $b
. That is why the result of the expression is also a double and is assigned to the variable $c
. Let's see another example.
// this variable is of type string
$a = "3";
// this variable is of type integer
$b = 2;
// the variable $c will be of type integer
$c = $a * $b;
echo gettype($c); // shows "integer"
PHP internally evaluates the variable $a
as integer to be able to multiply it with the variable $b
. That is why the result of the expression is also an integer and is assigned to the variable $c
. Let's evaluate a different context other than multiplication, for example concatenation.
// this variable is of type string
$text = "Resultado: ";
// this variable is of type integer
$number = 200;
$ans = $text . $number;
echo gettype($c) . "\n"; // shows "string"
echo $ans; // shows "Resultado: 200"
In this case, the variable $number
is converted to string to concatenate with $text
and give the result to the variable $ans
.