Dynamic classes in Vue.js.
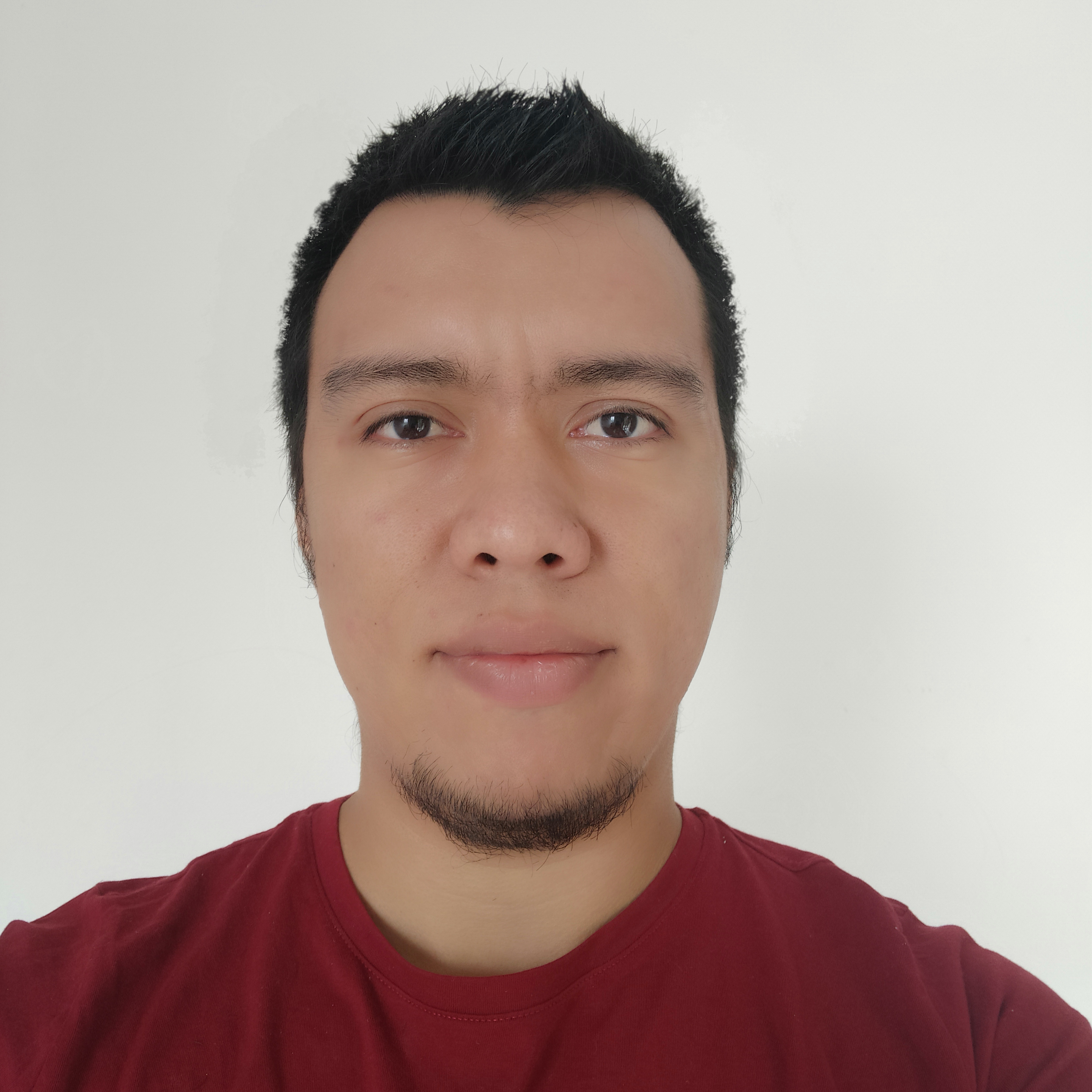
In Vue.js there is a very simple way to dynamically add classes to an HTML element. The way to do it is linked to how the v-bind directive works in Vue which we have previously discussed in the post Linking attributes in Vue.js.
For this example we will use the following CSS code.
.red {
color: red;
}
.green {
color: green;
}
.price {
letter-spacing: 4px;
}
And we will define the sales element with the following daily billing values.
var vue = new Vue({
el: "#app",
data: {
sales: {
'2020-01-01': 100,
'2020-01-02': 200,
'2020-01-03': 300
}
}
});
Suppose we want to show billing in red for orders of 100 USD or less, and in green for orders above 100 USD. For this, we can use the shortcut v-bind directive to place an object in the array attribute and decide if each class is applied:
<ul id="app">
<li class="price"
:class="{ red: price <= 100, green: price > 100 }"
v-for="(price, date) in sales">
{{ '\{\{ date \}\}' }} ____ $ {{ '\{\{ price \}\}' }}
</li>
</ul>
Note also that we have intentionally placed the price class on the li element. With this, we want to show that the v-bind directive attaches the selected class to those that already exist. Let's see the previous example in action with codepen.
Array Syntax
While it is possible to pass an object in the class attribute, it is also possible to pass an array of classes to add. Let's see a simple example where we attach a couple of classes to a button.
<button :class="['btn', 'btn-success']">Submit</button>
This is exactly the same as doing the following:
<button class="btn btn-primary">Submit</button>
At first glance it might not seem very useful, the true power of this syntax is that you can combine it with the object syntax. So it can be useful if you need to check if you really need the btn-success
class on a button or you need btn-danger
. That said, we could have something like the following:
<button
:class="['btn', isError ? 'btn-danger' : 'btn-success']"
@click="isError = !isError">Submit</button>
By clicking the button, the class will change every time depending on the value of isError. You can see this example running on codepen.