Expanded Widgets in Flutter
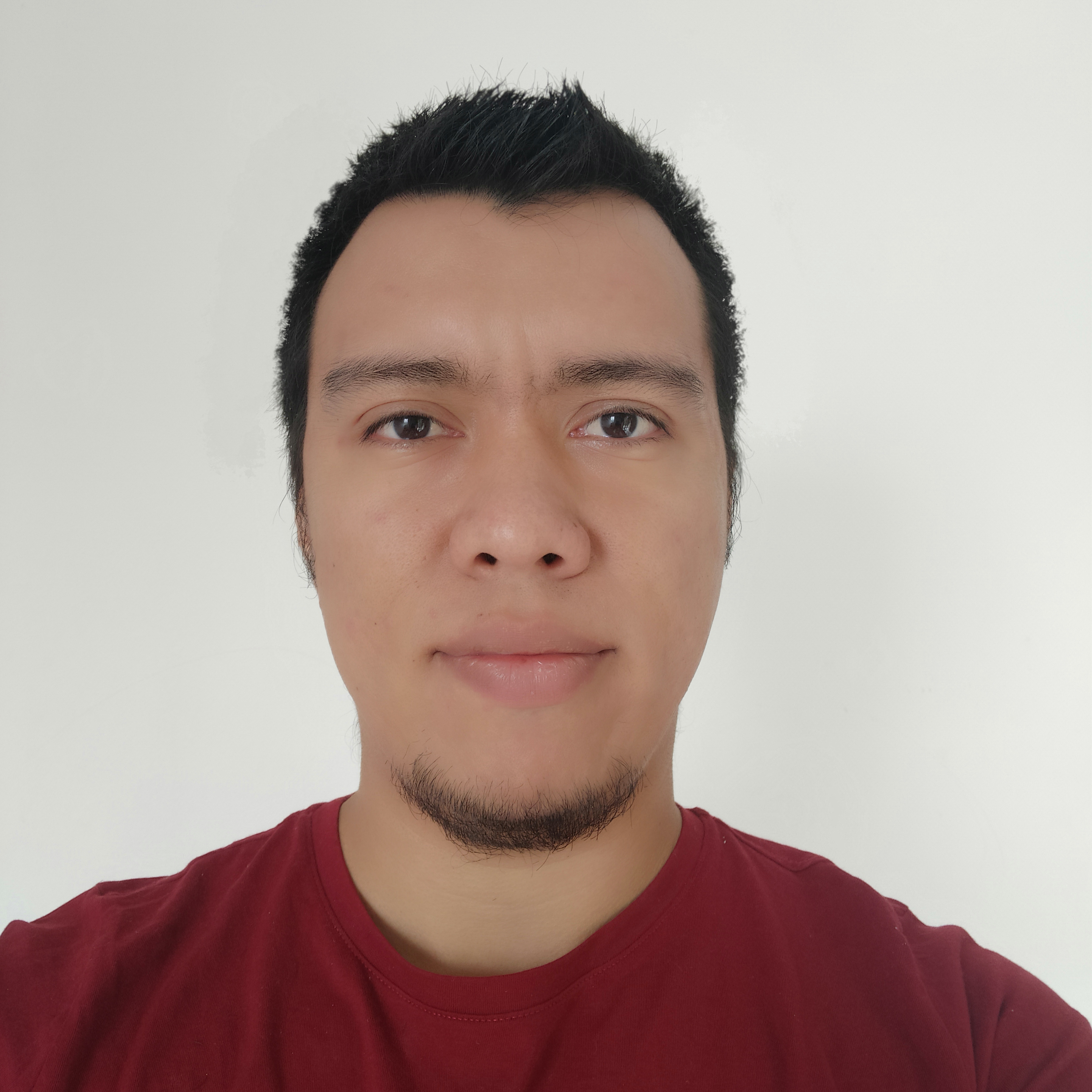
In a previous post we have seen two of the most important widgets for interface design, Row widget and Column widget. Today we will see a complement that will allow you to go a bit further in interface design with flex, the expanded widgets.
By default, widgets do not have the ability to expand, except for certain scenarios. However, if we want a widget to expand, we will have to explicitly indicate it. Let's see the following example where we have positioned three containers in a row.
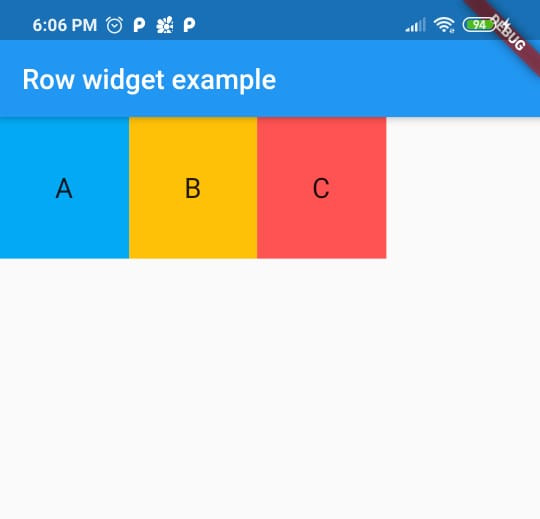
You can replicate this example using the following code to create the row with the three containers or see a complete example in the post Rows and Columns in Flutter.
Row(
children: [
Container(
padding: EdgeInsets.all(40),
color: Colors.lightBlue,
child: Text('A', style: TextStyle(fontSize: 20)),
),
Container(
padding: EdgeInsets.all(40),
color: Colors.amber,
child: Text('B', style: TextStyle(fontSize: 20)),
),
Container(
padding: EdgeInsets.all(40),
color: Colors.redAccent,
child: Text('C', style: TextStyle(fontSize: 20)),
),
],
),
Now imagine that what you really want is for the containers to take up all the available space in the row, that is, to expand. Something like what you see below.
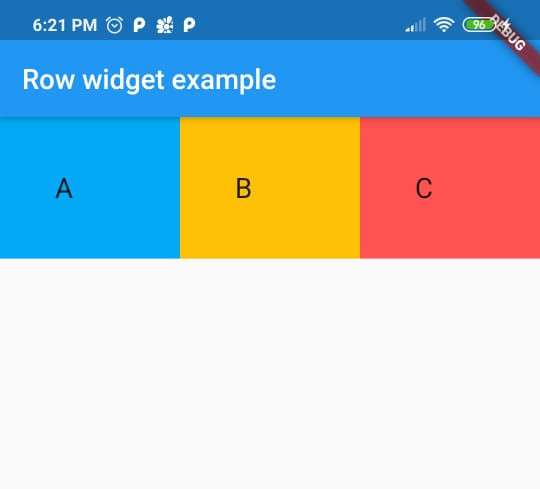
The solution is simple, use the Expanded
widget to make your widgets occupy the available space. Simply wrap each Container
inside this new widget like this:
Expanded(
child: Container(
padding: EdgeInsets.all(40),
color: Colors.lightBlue,
child: Text('A', style: TextStyle(fontSize: 20)),
),
)
This is very similar to how flex works in CSS. Now let's see what happens if we only expand the first widget.
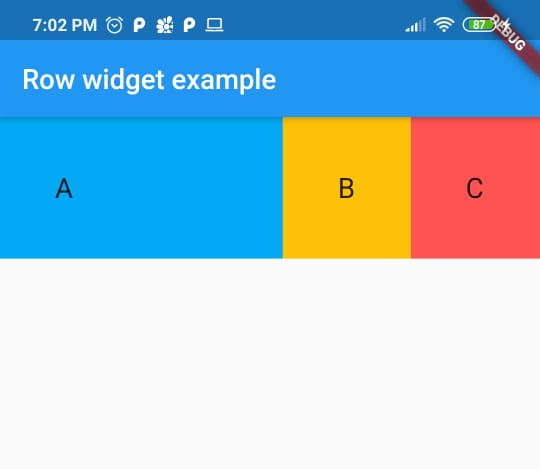
As you can see, at this point it is already intuitive to assume the result that expanding only the middle element will have.
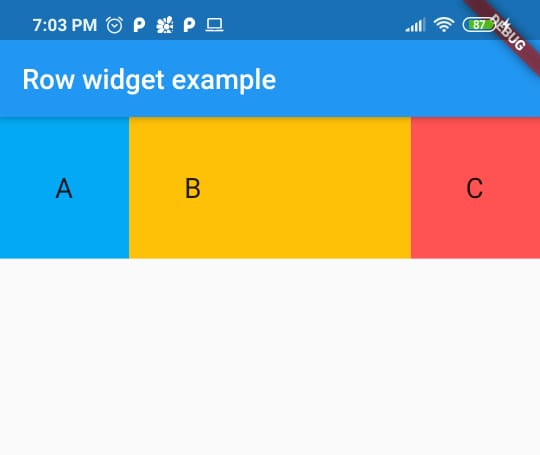
Finally, you can also modify the relationship each element will have with each other. If you wanted the elements to take up all the available space but be in a 1:2:4 ratio, you could use the flex property to achieve a result like the following:
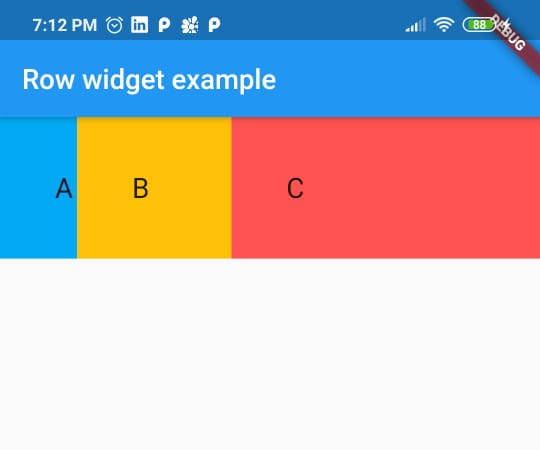
Row(
children: [
Expanded(
flex: 1,
child: Container(
padding: EdgeInsets.all(40),
color: Colors.lightBlue,
child: Text('A', style: TextStyle(fontSize: 20)),
),
),
Expanded(
flex: 2,
child: Container(
padding: EdgeInsets.all(40),
color: Colors.amber,
child: Text('B', style: TextStyle(fontSize: 20)),
),
),
Expanded(
flex: 4,
child: Container(
padding: EdgeInsets.all(40),
color: Colors.redAccent,
child: Text('C', style: TextStyle(fontSize: 20)),
),
),
],
)