Iteration Statements in Java (while, do-while)
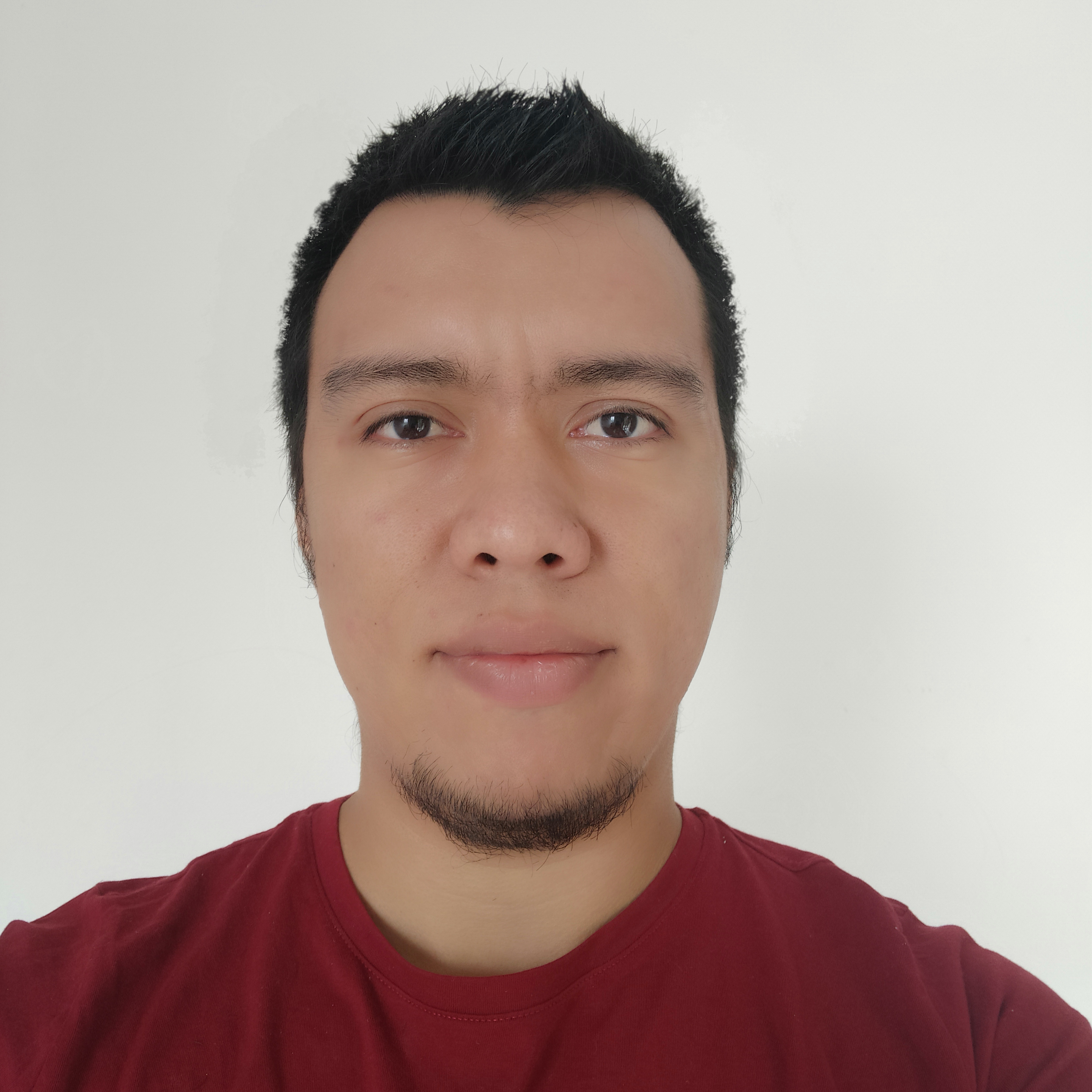
In a previous post of this series we have seen Decision Statements in Java (switch), today we will begin with the iterative statements of which we will see Do-While and While. Iterative statements allow you to execute a block of code as many times as necessary. Let's see how these statements work.
While Statement
This statement allows you to execute a block of code as many times as a statement is true. This implies that if at the beginning the statement is false then the block of code will not be executed even once. Let's see the following example.
public class Test
{
public static void main(String[] args) {
int counter = 1, until = 5;
while (counter <= until) {
System.out.println("Itaration #" + counter);
counter++;
}
}
}
Running this code produces an output like the following:
Itaration #1
Itaration #2
Itaration #3
Itaration #4
Itaration #5
As you can see, the same block of code has been executed five times, printing the respective iteration number. As in any loop, you must make sure that the cycle will end at some point, in this case in each iteration it is checked that the variable counter
is less than until
and the value of the variable is increased by one so that when it reaches five it is the last iteration.
Do-While Statement
This statement is similar to the while statement in that the block of code is executed as long as a statement is true. The big difference with respect to the previous one is that the block of code inside this statement is executed at least once, that is, the value of the statement is evaluated at the end. Let's see the following example.
public class Test
{
public static void main(String[] args) {
int a, b, ans;
String action;
Scanner input;
do {
System.out.println("Enter 'a' number: ");
input = new Scanner(System.in);
a = Integer.valueOf(input.nextLine());
System.out.println("Enter 'b' number: ");
input = new Scanner(System.in);
b = Integer.valueOf(input.nextLine());
ans = a + b;
System.out.println(String.valueOf(a) + " + " + String.valueOf(b) + " = " + String.valueOf(a+b));
System.out.println("Do you want to calculate the sum of another two numbers ? (Y/N)");
Scanner teclado = new Scanner(System.in);
action = teclado.nextLine();
} while (action.equals("Y"));
}
}
The output will be similar to the following.
Enter 'a' number:
14
Enter 'b' number:
16
14 + 16 = 30
Do you want to calculate the sum of another two numbers ? (Y/N)
N
...Program finished with exit code 0
Press ENTER to exit console
Let's observe this program in detail. In the first three lines of the main
method, some program variables are declared. Following this, the block of code within the curly braces prompts the user to enter two values, a and b, via console. After this, the sum of these two values is performed and the result is printed. The last three lines inside the braces ask the user whether to calculate the sum again and store what was typed in the action
variable. Finally, the condition is evaluated, if the user entered the character Y then the whole block will be executed again. As you see, in the end the block is executed at least once.