Sending data from a child component to a parent component in Vue.js.
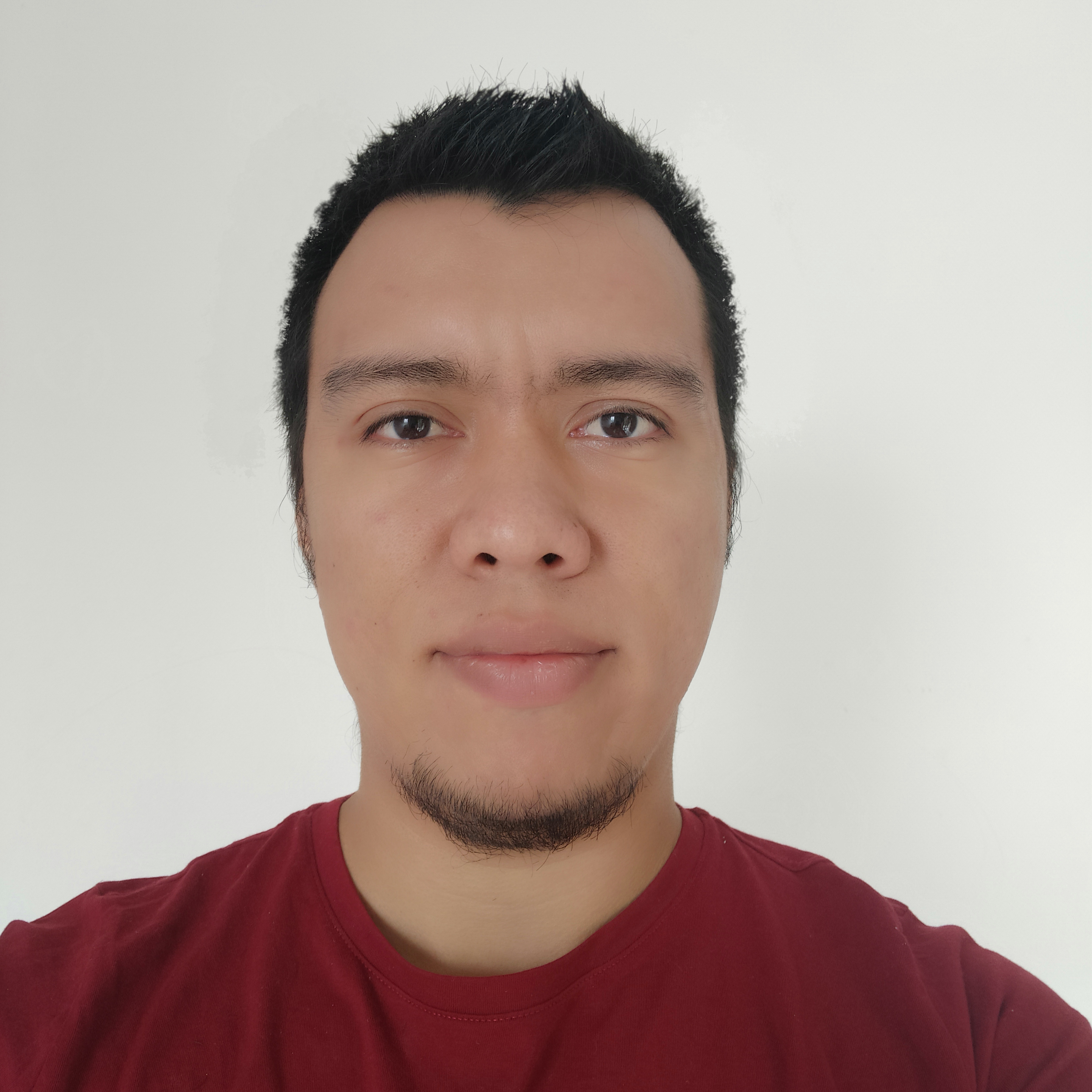
In a previous post we saw How to Create a Component in Vue.js and how to pass parameters to a component in Vue from the main instance. Today we'll see how to do the opposite, sending parameters or information from a child component to the parent.
To pass information to a parent component, we must first know how the communication between components in Vue works. In the previously mentioned post, we saw that it is possible to send parameters to components thanks to the props object. However, a component communicates with its parent through events. These events are emitted through the $emit
method.
$emit Method in Vue.js
With the method $emit we can launch an event created by ourselves to be captured in the component's declaration. To understand this, let's suppose we have a form in our HTML code and a submit button in a component outside of said form. What we want to do is to have said button send an event that changes the state of the form and adds a class called loading.
<div id="app" class="m-3">
<form :class="formClass">
<div class="form-group">
<label for="email">Email address</label>
<input type="email" class="form-control" id="email">
</div>
</form>
<x-button
text="Submit"
v-on:submit-form="formClass = 'loading'"></x-button>
</div>
As you can see, we are using the attribute binding to make a bind of the form's class with a property called formClass
. On the other hand, outside the form we have a component called x-button which will listen to an event called submit-form
that will change the value of the formClass property to loading.
Now, let's take a look at the component's definition.
Vue.component('x-button', {
props: ['text'],
template: `
<button type="submit" class="btn btn-primary" v-on:click="$emit('submit-form')">
{{ '\{\{ text \}\}' }}
</button>
`
});
var vue = new Vue({
el: "#app",
data: {
formClass: ''
}
})
Notice that in the definition of our component's template we have used the $emit('submit-form')
method to launch said event when the button is clicked. This event will be listened to by x-button and will change the form's class as previously mentioned. Let's see this example in action on Codepen.
Finally, to pass data from the child component to the parent, we just need to send more parameters to the $emit
method. In this case, we can directly pass the name of the class we want to assign to the form.
v-on:click="$emit('submit-form', 'loading')"
On the other hand, to be able to receive this parameter we must create a method in the Vue instance like this:
methods: {
changeFormState: function(className) {
this.formClass = className;
}
}
For this to work we must also change the expression in the event.
<x-button
text="Submit"
v-on:submit-form="changeFormState"></x-button>
The result will be exactly the same as before, with the difference that we have passed the name of the class's data from the child component to the parent.