List in Flutter
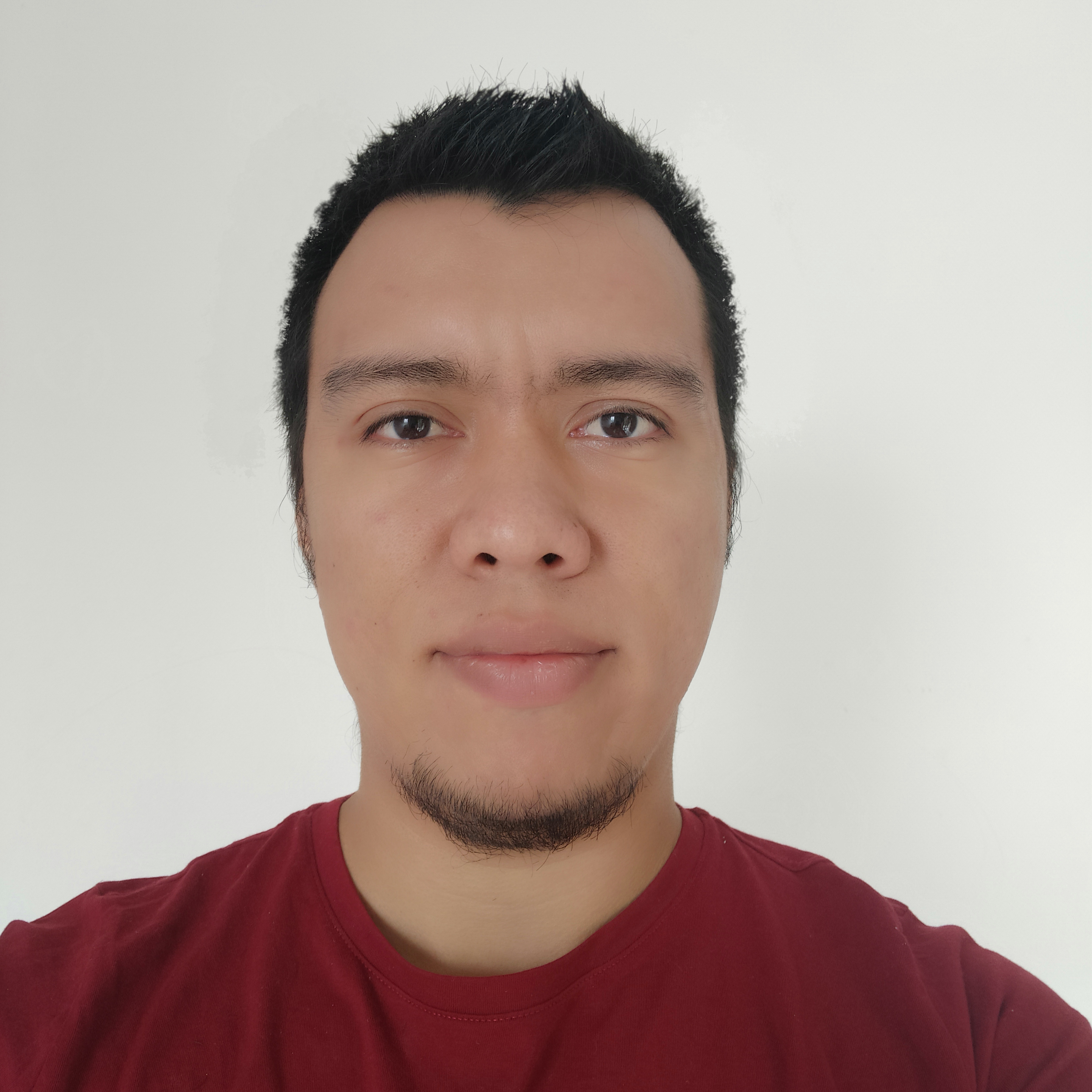
The List
class represents an iterable collection of objects. With this class we can save several objects of the same type and use all the methods that List brings natively to traverse collections and perform transformations on each element.
List Definition
To define the iterable collection of elements we can use the List
class followed by the data type that the list will contain.
List<type> varName = [
var1,
var2,
...
];
Traversing a List of Strings
Suppose we have the following list of strings:
List quotes = [
'The greatest glory in living lies not in never falling, but in rising every time we fall.',
'The way to get started is to quit talking and begin doing.',
'If life were predictable it would cease to be life, and be without flavor.'
];
To traverse this list, we just need to use the map
method in a very similar way to how we would use it in JavaScript.
Column(
children: quotes.map((text) {
return Container(
padding: EdgeInsets.all(20),
child: Text(
text,
style: TextStyle(
fontSize: 25,
color: Colors.green
),
)
);
}).toList()
)
Note that we have used the toList()
method to return the list of widgets needed by the Column
children property. The result would be similar to the following:
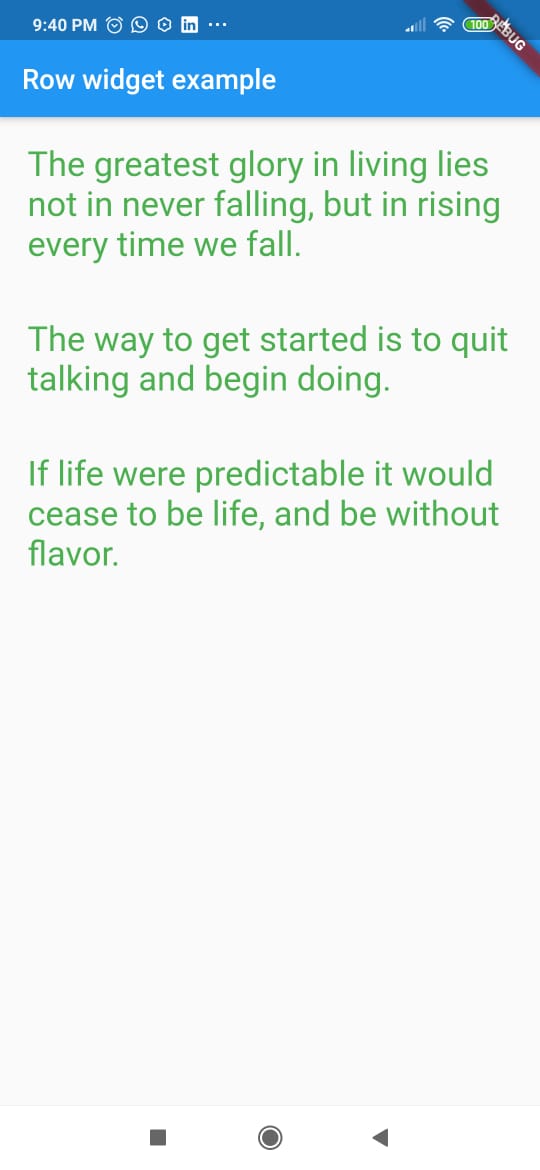
Traversing a List of Objects
Suppose we have the following Quote
class.
class Quote {
String text;
String author;
Quote({ this.text, this.author });
}
To iterate this class, we must first define the list in reference to this data type as follows:
List quotes = [
Quote(author: 'Nelson Mandela', text: 'The greatest glory in living lies not in never falling, but in rising every time we fall.'),
Quote(author: 'Walt Disney', text: 'The way to get started is to quit talking and begin doing.'),
Quote(author: 'Eleanor Roosevelt', text: 'If life were predictable it would cease to be life, and be without flavor.')
];
After this, we must use the properties of the Quote
object.
Column(
children: quotes.map((quote) {
return Container(
padding: EdgeInsets.all(20),
child: Text(
'${quote.text} - ${quote.author}',
style: TextStyle(
fontSize: 25,
color: Colors.green
),
)
);
}).toList()
The result would be as follows.
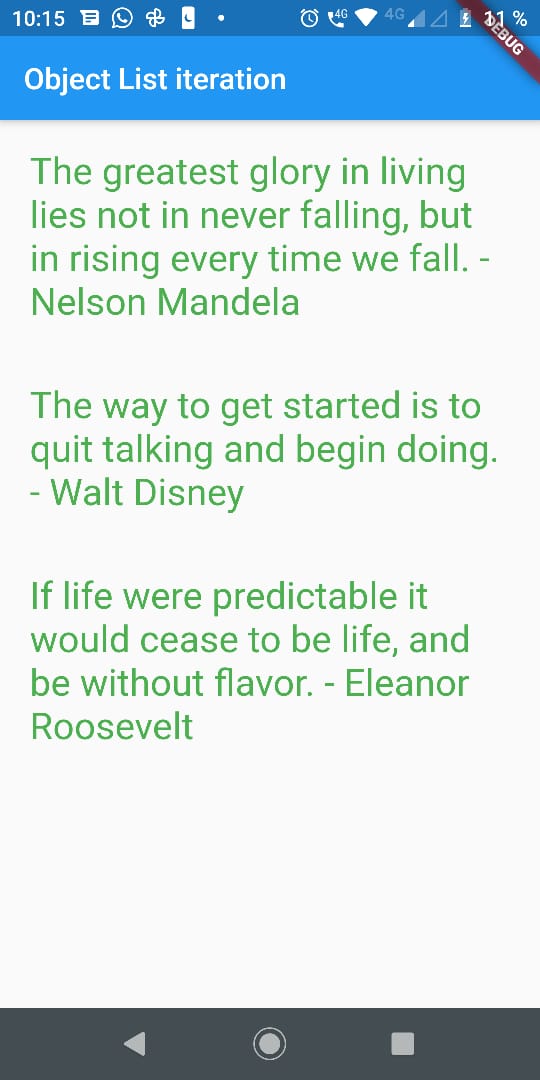